C#怎么使用C++的函数
时间: 2024-01-19 21:03:52 浏览: 73
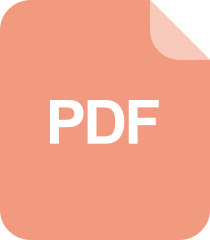
c++函数转c#函数示例程序分享
在 C# 中使用 C++ 函数有几种方式:
1. 使用平台调用(P/Invoke):
可以通过声明 extern 关键字和 DllImport 特性来引入 C++ 函数,并在 C# 中调用它们。首先,需要在 C++ 中将函数声明为 `extern "C"`,以确保使用 C 语言的约定。然后,在 C# 中使用 `DllImport` 特性指定 C++ 函数所在的动态链接库(DLL)以及函数的名称和签名。
示例代码:
```c++
// C++ DLL
extern "C" __declspec(dllexport) int Add(int a, int b)
{
return a + b;
}
```
```c#
// C# P/Invoke
using System;
using System.Runtime.InteropServices;
class Program
{
[DllImport("YourCppDll.dll")]
public static extern int Add(int a, int b);
static void Main(string[] args)
{
int result = Add(2, 3);
Console.WriteLine(result); // 输出 5
}
}
```
2. 使用 C++/CLI(Managed C++):
C++/CLI 是一种混合语言,可以在其中同时使用 C++ 和 C#。通过创建一个 C++/CLI 类,可以将 C++ 函数包装为公共成员,并在 C# 中直接调用该类的方法。
示例代码:
```cpp
// C++/CLI Wrapper
#pragma managed
public ref class MyCppWrapper
{
public:
static int Add(int a, int b)
{
return a + b;
}
};
```
```c#
// C# 使用 C++/CLI Wrapper
class Program
{
static void Main(string[] args)
{
int result = MyCppWrapper.Add(2, 3);
Console.WriteLine(result); // 输出 5
}
}
```
无论使用哪种方法,都需要确保 C++ 函数的可访问性和适当的函数签名,以便在 C# 中正确调用。
阅读全文
相关推荐
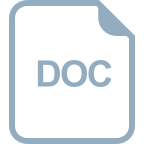
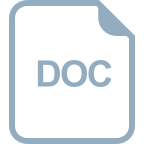
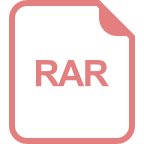
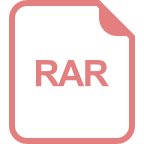
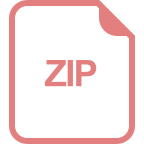
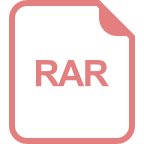
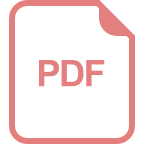
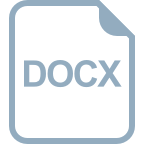
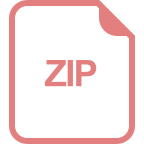
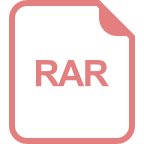
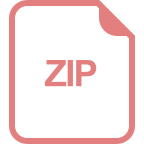
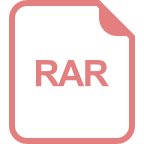
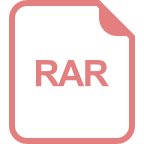
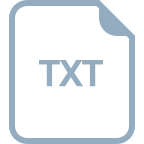
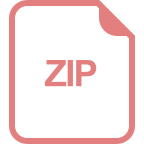