React hooks 用法
时间: 2024-09-09 13:02:53 浏览: 45
React Hooks 是 React 16.8 版本引入的新特性,它允许你在不编写类组件的情况下使用 state 和其他 React 特性。Hooks 提供了一种新的方式,让你可以在函数组件内部使用 React 的特性。以下是 React Hooks 的一些基本用法:
1. useState
`useState` 是最常用的 Hook,它用于为函数组件添加 state。它接收一个初始值,并返回一个数组,其中第一个元素是当前的 state,第二个元素是一个函数用于更新 state。
```javascript
import React, { useState } from 'react';
function Example() {
// 声明一个叫 "count" 的 state 变量
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
```
2. useEffect
`useEffect` 用于处理副作用。在组件渲染之后执行一些操作,比如发送网络请求或更新 DOM。
```javascript
import React, { useState, useEffect } from 'react';
function Example() {
const [count, setCount] = useState(0);
// 相当于 componentDidMount 和 componentDidUpdate:
useEffect(() => {
// 更新文档的标题
document.title = `You clicked ${count} times`;
});
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
```
3. useContext
`useContext` 允许你订阅 React 的 context,以避免在组件树中手动传递 props。
```javascript
import React, { useContext } from 'react';
import { MyContext } from './MyContext';
function MyComponent() {
const value = useContext(MyContext);
return (
<div>
useContext value: {value}
</div>
);
}
```
4. 自定义 Hooks
你还可以通过组合现有的 Hooks 创建自己的 Hooks,以便在多个组件之间重用状态逻辑。
```javascript
import React, { useState, useEffect } from 'react';
function useMousePosition() {
const [position, setPosition] = useState({ x: 0, y: 0 });
useEffect(() => {
const handleMove = e => {
setPosition({ x: e.clientX, y: e.clientY });
};
window.addEventListener('mousemove', handleMove);
// 清理副作用
return () => {
window.removeEventListener('mousemove', handleMove);
};
}, []);
return position;
}
function MyComponent() {
const position = useMousePosition();
return (
<div>
The mouse position is {position.x}, {position.y}
</div>
);
}
```
阅读全文
相关推荐
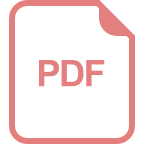
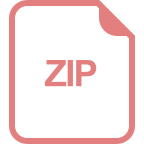
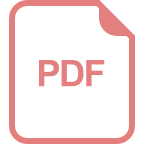
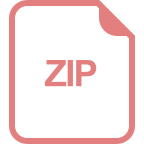
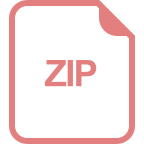
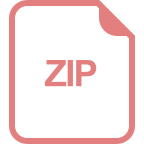
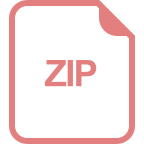
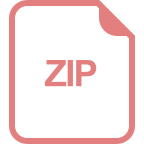
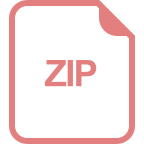
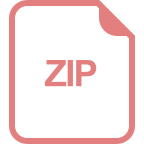
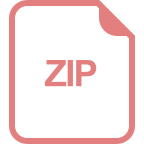
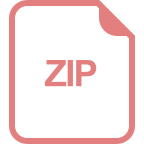
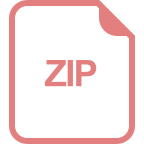
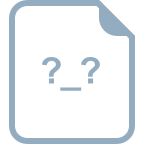
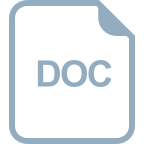



