普通附合水准路线,已知起始点和终点,测站数已知,用C#编写程序
时间: 2024-09-11 09:06:15 浏览: 35
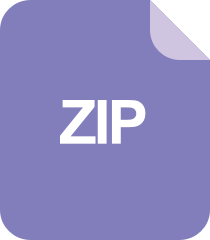
附合水准路线间接平差计算.zip

在C#中,要编写一个计算普通附合水准路线的程序,首先需要了解一些基本的概念,如高程测量、距离计算以及线路的节点数据。以下是一个简单的示例,假设我们有起始点、终点的坐标(可以是经纬度或其他坐标系统),以及固定的测站数。我们将创建一个类来表示路线,并包含必要的函数来进行计算。
```csharp
using System;
class RouteCalculator
{
// 定义一个节点类,包含经纬度信息
public class Node
{
public double Longitude { get; set; }
public double Latitude { get; set; }
}
// 起始点和终点
private Node startNode;
private Node endNode;
private int stationCount;
public RouteCalculator(Node start, Node end, int stations)
{
startNode = start;
endNode = end;
stationCount = stations;
}
// 理想情况下,这里应该通过真实的地理API获取每个站点的距离,这里仅作为示例
private double GetDistance(Node from, Node to)
{
// 这里只是一个伪距离计算,实际应用应使用经纬度计算库
return Math.Sqrt(Math.Pow(to.Longitude - from.Longitude, 2) + Math.Pow(to.Latitude - from.Latitude, 2));
}
// 计算整个路线的总长度
public double CalculateTotalLength()
{
double length = 0;
for (int i = 0; i < stationCount; i++)
{
if (i == 0) // 起始点到第一个测站
length += GetDistance(startNode, stations[i]);
else // 后续各测站之间的距离
length += GetDistance(stations[i - 1], stations[i]);
}
length += GetDistance(stations[stationCount - 1], endNode); // 最后一站到终点
return length;
}
}
// 使用示例
public static void Main(string[] args)
{
Node start = new Node() { Longitude = 116.407, Latitude = 39.904 };
Node end = new Node() { Longitude = 116.390, Latitude = 39.915 };
var route = new RouteCalculator(start, end, 3); // 假设有3个测站
double totalLength = route.CalculateTotalLength();
Console.WriteLine($"普通附合水准路线总长度: {totalLength} 单位(例如米)");
}
```
阅读全文
相关推荐
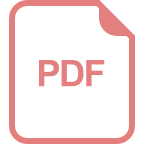
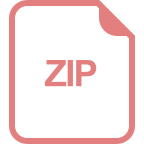






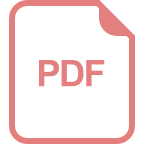
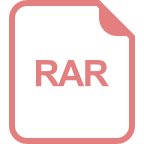
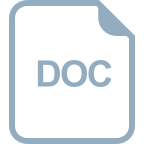


