ffmpeg使用api库将mp4转成mkv和wav文件
时间: 2024-11-23 15:49:32 浏览: 22
FFmpeg是一个强大的命令行工具,用于处理音频、视频流和其他多媒体数据,它也提供了API库供开发者使用。如果你想通过API库而不是命令行工具将MP4转换为MKV和WAV文件,你可以利用FFmpeg的libavcodec、libavformat和libavfilter库。
以下是一个简单的示例,展示如何使用C++的FFmpeg API进行转换:
```cpp
#include <ffplay.h> // 包含FFmpeg的C++接口
int main() {
AVFormatContext* input_ctx = nullptr;
AVFormatContext* output_ctx MKV = nullptr;
AVStream* audio_stream = nullptr;
AVCodecContext* codec_ctx = nullptr;
// 打开输入MP4文件
if (avformat_open_input(&input_ctx, "input.mp4", NULL, NULL) != 0) {
// 错误处理
return -1;
}
// 检查是否成功打开
if (!input_ctx) {
// 错误处理
return -1;
}
// 查找音频流
for (unsigned int i = 0; i < avformat_get_n Streams(input_ctx); ++i) {
if (strcmp(avstream_get_codec_name(input_ctx->streams[i]), "aac") == 0 || // 检查AAC编码
strcmp(avstream_get_codec_name(input_ctx->streams[i]), "mp3") == 0) { // 或者其他音频编码
audio_stream = input_ctx->streams[i];
break;
}
}
// 创建输出MKV和WAV文件上下文
if (!(output_ctx = avformat_alloc_context())) {
// 错误处理
return -1;
}
output_ctx->oformat = av_guess_format("mkv", NULL, NULL);
if (!(codec_ctx = avcodec_alloc_context3(NULL))) {
// 错误处理
return -1;
}
// 将音频流复制到输出文件
if (avcodec_copy_context(codec_ctx, audio_stream->codec) != 0) {
// 错误处理
return -1;
}
codec_ctx->codec_type = AVMEDIA_TYPE_AUDIO;
// 添加音频流到输出文件
if (avformat_new_stream(output_ctx, codec_ctx) < 0) {
// 错误处理
return -1;
}
// 编码并写入文件
if (avio_open(&output_ctx->pb, "output.mkv", AVIO_FLAG_WRITE | AVIO_FLAG_TRUNCATE) < 0) {
// 错误处理
return -1;
}
if (avcodec_encode_audio2(codec_ctx, &audio_frame, input_data, input_size) >= 0) {
av_interleaved_write_frame(output_ctx, &audio_frame);
}
// 同样的步骤可以为输出WAV文件重复,只需要调整文件格式和解码器设置。
// 关闭资源
avio_close(output_ctx->pb);
av_free(output_ctx);
avcodec_free_context(&codec_ctx);
return 0;
}
```
阅读全文
相关推荐












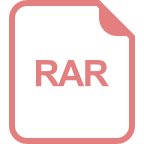


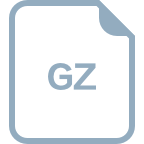


