opencv中使用最小包围圆圈出第四行第四列的小鸭子
时间: 2024-10-15 12:07:29 浏览: 24
在OpenCV中,要找出图像中小鸭子的最小包围圆形,通常需要经过以下几个步骤:
1. **读取和预处理图像**:
使用`cv2.imread()`函数读取图片,并对图像进行预处理,如调整大小、灰度化或二值化,以便于检测。
```python
import cv2
img = cv2.imread('duck_image.jpg')
gray_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
```
2. **边缘检测**:
可能需要用Canny算子或其他边缘检测算法来增强小鸭子轮廓的清晰度。
```python
edges = cv2.Canny(gray_img, threshold1, threshold2)
```
3. **找到轮廓**:
使用`cv2.findContours()`函数查找图像中的轮廓。这一步会返回轮廓列表和表示轮廓层次结构的信息。
```python
contours, _ = cv2.findContours(edges, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
```
4. **遍历轮廓**:
遍历所有轮廓,针对每个轮廓计算中心点并判断是否位于第四行第四列。可以使用`cv2.boundingRect()`获取轮廓的边界框信息。
```python
row, col = 4, 4
for contour in contours:
x, y, w, h = cv2.boundingRect(contour)
# 检查轮廓位置 (x, y) 是否满足条件
if x >= row * img.shape[1] and x < (row + 1) * img.shape[1] \
and y >= col * img.shape[0] and y < (col + 1) * img.shape[0]:
circle_center = (x + w // 2, y + h // 2) # 计算圆心坐标
# 继续计算最小包围圆
radius = cv2.minEnclosingCircle(contour)
center, radius = radius[0], radius[1]
```
5. **画出最小包围圆**:
如果找到了符合条件的轮廓,使用`cv2.circle()`画出最小包围圆。
```python
if circle_center is not None:
cv2.circle(img, circle_center, int(radius), (0, 255, 0), 2) # 绿色圆环
```
6. **显示结果**:
最后,使用`cv2.imshow()`展示处理后的图像。
```python
cv2.imshow("Detected Circle", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
阅读全文
相关推荐
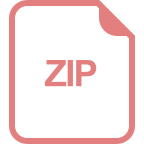
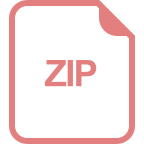
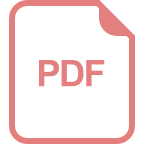
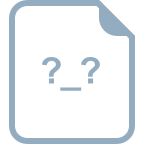
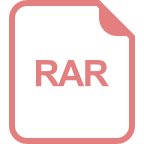
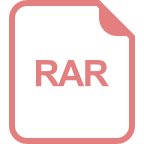
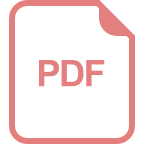
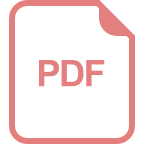
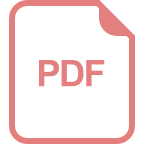
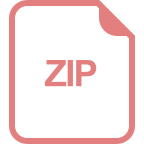
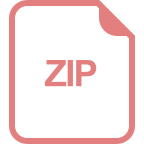
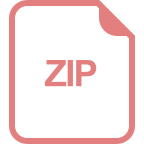
