请用Pyecharts绘制函数 f(x,y)=sin(sqrt(x**2+y**2))/sqrt(x**2+y**2)的三维图像
时间: 2024-12-16 16:12:18 浏览: 4
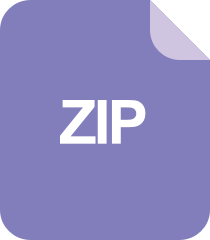
Sqrt(x^2 + y^2) 和 atan(y/x) 通过 CORDIC:将笛卡尔坐标 (x,y) 转换为极坐标 (r,theta)-matlab开发
在Pyecharts中,我们可以使用`Chart3D`类来创建3D图形,但是直接绘制复杂的数学函数并不内置在这个库中。不过,你可以先用其他工具如Matplotlib计算出数据点,然后将结果转换成Pyecharts可以处理的数据格式。这里我将提供一个基本的步骤:
首先,你需要安装matplotlib,如果还没有安装,可以使用pip命令:
```bash
pip install matplotlib numpy pyecharts
```
接下来,我们将使用matplotlib来生成数据,并使用Pyecharts显示三维图。这是一个示例代码:
```python
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
from pyecharts import options as opts
from pyecharts.charts import Chart3D
# 定义数学函数
def f(x, y):
return np.sin(np.sqrt(x**2 + y**2)) / np.sqrt(x**2 + y**2)
# 创建网格
x = np.linspace(-5, 5, 100)
y = np.linspace(-5, 5, 100)
X, Y = np.meshgrid(x, y)
Z = f(X, Y)
# 使用matplotlib制作3D图
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.plot_surface(X, Y, Z, cmap='viridis')
# 将matplotlib的3D图形转为Pyecharts的数据格式
data = [(x, y, z) for x, y, z in zip(X.ravel(), Y.ravel(), Z.ravel())]
# 创建并渲染Pyecharts的3D图表
chart3d = (
Chart3D()
.add_schema(
"3D Surface",
{
"xAxis": {"name": "X", "type": "category"},
"yAxis": {"name": "Y", "type": "category"},
"zAxis": {"name": "Z"},
}
)
.add("3D Surface", data, is_visualmap=True, visual_range=[-1, 1], visual_text_color="#fff")
.set_global_opts(title_opts=opts.TitleOpts(title="3D Function Plot"))
.render()
)
# 输出到HTML文件或浏览器
chart3d.show_config()
```
注意这只是一个基础示例,实际应用中可能需要调整颜色映射、视觉范围等细节。如果你想要在控制台展示动态效果,可能需要结合ECharts或其他可视化库。
阅读全文
相关推荐
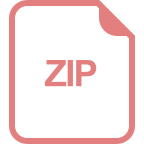
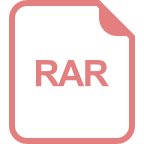
















