asp.netcore学生管理系统
时间: 2024-12-25 21:21:31 浏览: 5
### 使用 ASP.NET Core 构建学生管理系统
#### 创建项目结构
为了创建一个基于 ASP.NET Core 的学生信息系统,首先需要设置开发环境并初始化一个新的 Web 应用程序。推荐使用的集成开发环境 (IDE) 是 Visual Studio 2019 或更高版本[^2]。
```bash
dotnet new webapp -n StudentManagementSystem
cd StudentManagementSystem
```
这段命令会生成一个基础的 MVC 结构的应用程序模板,其中包含了控制器、视图以及模型文件夹来帮助组织代码逻辑[^1]。
#### 设计数据模型
定义实体类表示数据库中的表,比如 `Student` 类可以用来存储学生的个人信息:
```csharp
public class Student
{
public int Id { get; set; }
public string Name { get; set; }
public DateTime BirthDate { get; set; }
}
```
接着配置 Entity Framework Core 来管理这些对象与关系型数据库之间的映射。
#### 实现业务逻辑层
通过编写服务接口和服务实现分离关注点,使应用程序更易于维护和测试。例如,创建 IStudentService 接口及其默认实现 DefaultStudentService:
```csharp
// 定义接口
public interface IStudentService {
Task<IEnumerable<Student>> GetAllStudentsAsync();
}
// 提供具体实现
public class DefaultStudentService : IStudentService {
private readonly ApplicationDbContext _context;
public DefaultStudentService(ApplicationDbContext context){
_context = context;
}
public async Task<IEnumerable<Student>> GetAllStudentsAsync(){
return await _context.Students.ToListAsync();
}
}
```
这有助于遵循依赖注入原则,并促进单元测试。
#### 配置路由与控制器
在 Controllers 文件夹下新增 StudentsController.cs 控制器用于处理 HTTP 请求并将响应返回给客户端浏览器。这里展示了一个简单的 GET 方法获取所有学生列表的例子:
```csharp
[ApiController]
[Route("[controller]")]
public class StudentsController : ControllerBase {
private readonly IStudentService studentService;
public StudentsController(IStudentService service){
this.studentService = service;
}
[HttpGet]
public async Task<IActionResult> Get() => Ok(await studentService.GetAllStudentsAsync());
}
```
上述代码片段展示了 RESTful API 的设计方式,它允许前端应用轻松调用后端资源。
#### 前端界面搭建
对于用户交互部分,则可以在 Views/Shared/_Layout.cshtml 中统一页面样式;而在 Views/Home/Index.cshtml 设置主页内容显示区域。此外还可以借助 Razor Pages 技术简化某些场景下的操作流程[^3]。
---
阅读全文
相关推荐
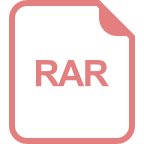
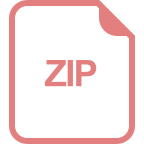
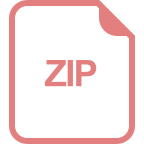
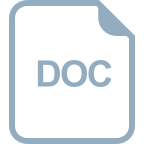
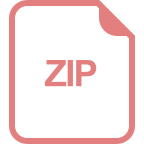
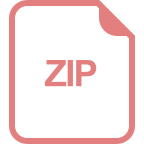
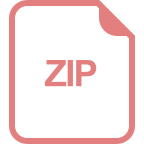
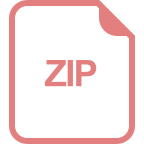
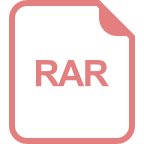
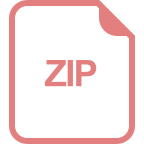
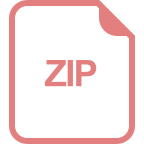
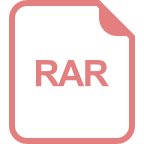
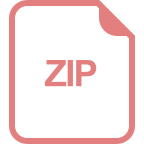
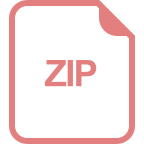
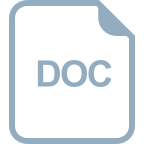
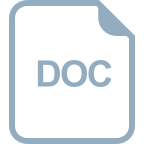
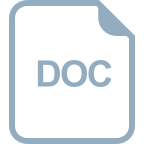
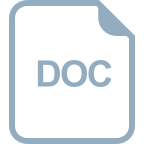