c语言写一个 一位数组10个元素中寻找重复最多次的值的程序
时间: 2024-09-26 18:12:39 浏览: 25
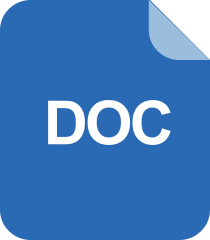
100个经典C语言程序源代码.doc
在 C 语言中,要编写一个程序来找出一个包含10个元素的一维整数数组中重复最多的数值,我们可以使用哈希表(如 C 语言中的 `unordered_map` 或者自定义的数据结构)来存储每个元素及其出现的次数。下面是一个简单的示例,使用了 `stdio.h`、`stdlib.h` 和 `string.h` 预处理指令:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define ARRAY_SIZE 10
typedef struct {
int value;
int count;
} Element;
Element* find_most_frequent(int arr[], int n) {
Element elements[ARRAY_SIZE] = {0};
int max_count = 0, max_index = -1;
// 初始化所有元素的计数为0
for (int i = 0; i < ARRAY_SIZE; ++i) {
elements[i].value = i;
elements[i].count = 0;
}
// 遍历数组,更新元素计数
for (int i = 0; i < n; ++i) {
int index = arr[i];
elements[index].count++;
// 如果当前计数超过最大计数,更新最大计数和索引
if (elements[index].count > max_count) {
max_count = elements[index].count;
max_index = index;
}
}
return &elements[max_index]; // 返回重复次数最多的元素
}
int main() {
int arr[ARRAY_SIZE] = {5, 2, 3, 2, 7, 1, 2, 8, 9, 2}; // 示例数组
int n = sizeof(arr) / sizeof(arr[0]);
Element* result = find_most_frequent(arr, n);
printf("重复次数最多的数字是 %d,出现了 %d 次.\n", result->value, result->count);
free(result); // 释放动态分配的内存
return 0;
}
```
在这个程序中,我们首先初始化一个大小为10的 `Element` 结构体数组,用于存储元素值和它们出现的次数。然后遍历输入数组,每次遇到一个元素就更新其计数,并检查是否超过了当前的最大计数。最后返回重复次数最多的元素。
阅读全文
相关推荐
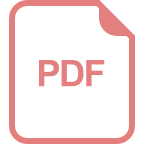
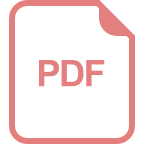

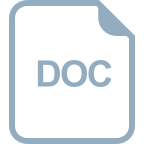
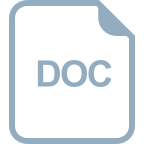
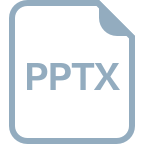
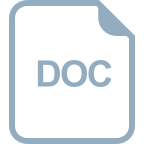
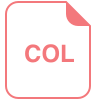
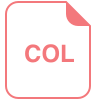


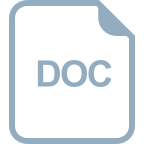
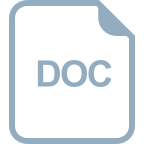
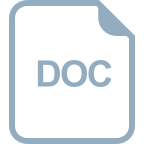
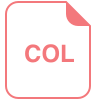
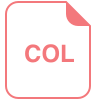