Linux系统用c语言获取系统间
时间: 2024-04-06 11:04:24 浏览: 100
你可以使用C语言中的系统调用来获取Linux系统的时间。具体来说,你可以使用time函数来获取当前的系统时间,或者使用ctime函数将时间转换为一个可读的字符串。以下是一个简单的示例代码:
```c
#include <stdio.h>
#include <time.h>
int main() {
time_t t = time(NULL);
struct tm *tm = localtime(&t);
printf("Current system time: %s", ctime(&t));
printf("Year: %d\n", tm->tm_year + 1900);
printf("Month: %d\n", tm->tm_mon + 1);
printf("Day: %d\n", tm->tm_mday);
printf("Hour: %d\n", tm->tm_hour);
printf("Minute: %d\n", tm->tm_min);
printf("Second: %d\n", tm->tm_sec);
return 0;
}
```
运行上述代码,你将会得到当前系统的日期和时间,以及其他详细信息。请注意,`localtime`函数会将时间转换为本地时间,因此结果可能因时区而异。
相关问题
linux中使用C语言获取Tick时钟
在linux中,可以使用函数`clock_gettime()`来获取系统运行时间。具体实现方法如下:
```c
#include <time.h>
uint64_t get_tick()
{
struct timespec ts;
clock_gettime(CLOCK_MONOTONIC_RAW, &ts);
return (uint64_t)ts.tv_sec * 1000000000 + ts.tv_nsec;
}
```
其中,`CLOCK_MONOTONIC_RAW`表示获取系统运行时间,可以保证时间单调递增。函数返回一个64位整数表示纳秒数。
linux下C语言获取系统版本信息
在Linux环境下,C语言可以使用标准库函数`uname()`来获取系统的版本信息。这个函数通常位于`<sys/uname.h>`头文件中。下面是一个简单的示例:
```c
#include <stdio.h>
#include <sys/uname.h>
int main() {
struct uname system_info;
int result;
// 调用uname()函数获取系统信息
result = uname(&system_info);
if (result == 0) { // 检查调用是否成功
printf("系统名称: %s\n", system_info.sysname); // 系统名称,如"Linux"
printf("内核版本: %s\n", system_info.release); // 内核版本,如"4.19.0-8-amd64"
printf("机器架构: %s\n", system_info.machine); // 机器架构,如"x86_64"
// 更详细的信息可以通过system_info.nodename、system_info.version、system_info.domaintype等字段获取
} else {
printf("获取系统信息失败\n");
}
return 0;
}
```
阅读全文
相关推荐
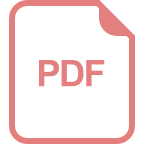
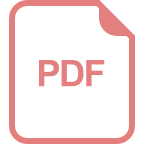
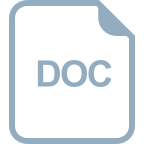
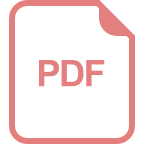
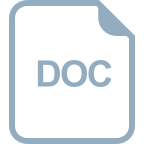
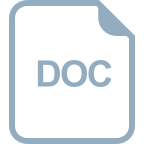
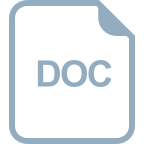
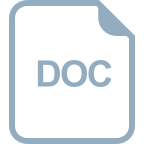
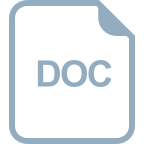
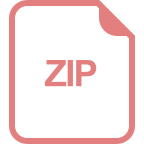
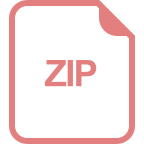