帮我用c语言单链表写一份图书管理系统代码
时间: 2024-09-11 22:10:05 浏览: 51
使用C语言编写一个单链表实现的图书管理系统,我们需要定义几个基本的数据结构和函数来完成这个系统。下面是一个简单的实现框架:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义图书结构体
typedef struct Book {
char title[50];
char author[50];
int id;
struct Book *next;
} Book;
// 创建新书节点
Book* createBook(int id, const char* title, const char* author) {
Book* newBook = (Book*)malloc(sizeof(Book));
if (newBook == NULL) {
printf("内存分配失败!\n");
return NULL;
}
strcpy(newBook->title, title);
strcpy(newBook->author, author);
newBook->id = id;
newBook->next = NULL;
return newBook;
}
// 向链表中添加图书
void addBook(Book **head, int id, const char* title, const char* author) {
Book *newBook = createBook(id, title, author);
newBook->next = *head;
*head = newBook;
}
// 显示所有图书信息
void displayBooks(Book *head) {
Book *current = head;
while (current != NULL) {
printf("ID: %d\nTitle: %s\nAuthor: %s\n\n", current->id, current->title, current->author);
current = current->next;
}
}
// 删除图书
void deleteBook(Book **head, int id) {
Book *current = *head;
Book *previous = NULL;
while (current != NULL && current->id != id) {
previous = current;
current = current->next;
}
if (current == NULL) {
printf("找不到ID为 %d 的图书。\n", id);
return;
}
if (previous == NULL) {
*head = current->next;
} else {
previous->next = current->next;
}
free(current);
}
// 清空图书链表
void clearBooks(Book **head) {
Book *current = *head;
Book *next;
while (current != NULL) {
next = current->next;
free(current);
current = next;
}
*head = NULL;
}
// 主函数
int main() {
Book *head = NULL; // 初始化链表头指针
// 添加图书
addBook(&head, 1, "C程序设计语言", "K&R");
addBook(&head, 2, "C和指针", "Kernighan & Ritchie");
addBook(&head, 3, "C专家编程", "Peter van der Linden");
// 显示所有图书
displayBooks(head);
// 删除图书
deleteBook(&head, 2);
// 再次显示所有图书
displayBooks(head);
// 清空图书链表
clearBooks(&head);
return 0;
}
```
这个代码是一个非常基础的图书管理系统的实现,它包含了添加图书、显示图书、删除图书和清空图书列表的功能。在实际应用中,你可能还需要添加查找图书、修改图书信息、保存和加载图书数据到文件等功能。此外,为了保证系统的健壮性和用户体验,错误处理和用户交互也是必须要考虑的方面。
阅读全文
相关推荐
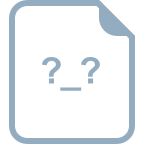
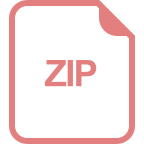
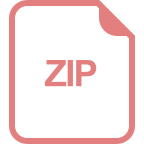
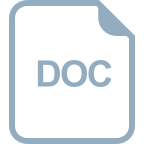
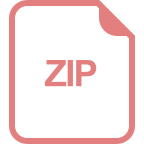
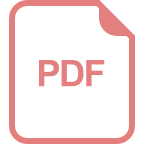
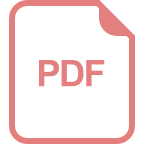
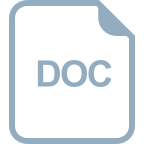
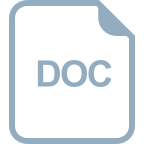
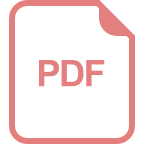
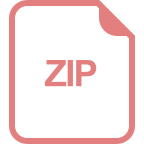
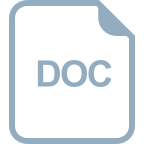
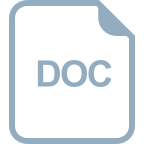
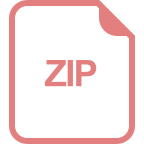
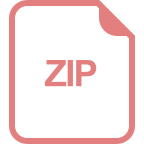