Springboot大数据量导出excel
时间: 2024-09-10 10:17:44 浏览: 91
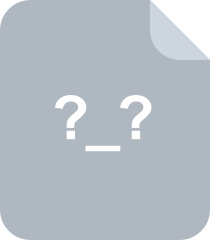
Java springboot简单实现excel表导出导入.md
Spring Boot 中的大数据量导出 Excel 通常通过 Apache POI 或者其他的库如 SheetXLSXWriter 实现。这个过程通常涉及以下几个步骤:
1. 引入依赖:首先,在你的 Maven 或 Gradle 项目中添加相关的 Excel 库依赖,比如 `spring-boot-starter-web` 和 `poi` 或 `xlsx-writer`。
```xml
<!-- Maven -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
</dependency>
// 或者 Gradle
implementation 'org.springframework.boot:spring-boot-starter-web'
implementation 'org.apache.poi:poi-ooxml'
```
2. 创建服务:创建一个服务类,利用 Java 的流式处理技术(如 Stream API)来读取数据并逐行写入 Excel 文件。例如:
```java
@Service
public class ExportService {
@Autowired
private YourRepository yourRepository;
public ResponseEntity<ByteArrayResource> exportToExcel() throws IOException {
List<DataModel> dataList = yourRepository.findAll();
try (OutputStream outputStream = new ByteArrayOutputStream()) {
XSSFWorkbook workbook = new XSSFWorkbook();
XSSFSheet sheet = workbook.createSheet("Sheet Name");
// 写入表头
for (String header : headers) {
Row row = sheet.createRow(0);
Cell cell = row.createCell(0);
cell.setCellValue(header);
}
// 写入数据
dataList.stream()
.map(rowData -> {
Row newRow = sheet.createRow(sheet.getLastRowNum() + 1);
rowData.forEach((key, value) -> newRow.createCell(keyIndex).setCellValue(value));
return newRow;
})
.forEach(Row::createCell);
workbook.write(outputStream);
outputStream.flush();
ByteArrayResource resource = new ByteArrayResource(outputStream.toByteArray());
resource.setFilename("export.xlsx");
return ResponseEntity.ok().contentType(MediaType.APPLICATION_OCTET_STREAM).body(resource);
}
}
}
```
这里假设 `yourRepository` 是一个查询大量数据的 Repository 接口,`headers` 是列名数组,`DataModel` 是你的实体类。
阅读全文
相关推荐
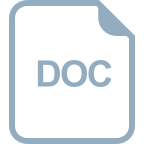
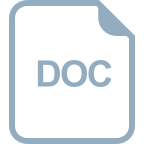
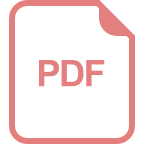
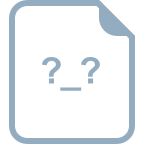
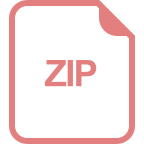
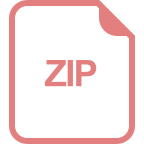
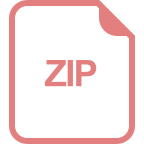
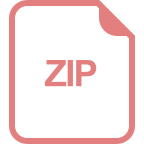
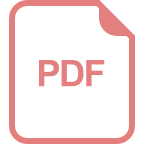
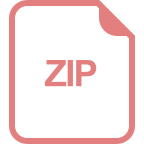
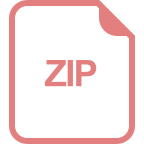
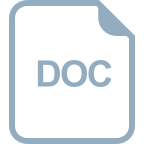
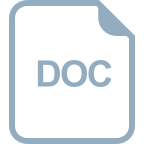


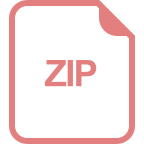
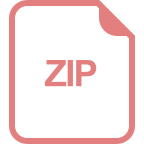
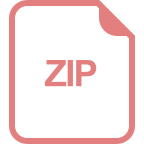