用C语言头插法创建一个单链表,从键盘输入数据,数字或者是字符
时间: 2024-09-26 16:02:03 浏览: 36
在C语言中,我们可以使用结构体定义链表节点,并利用头插法来动态地从键盘输入数据(数字或字符)创建单链表。下面是一个简单的示例程序:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
typedef struct Node {
int data; // 如果是整数
char data_char; // 如果是字符
struct Node* next;
} Node;
// 创建新节点函数
Node* create_node(int num 或者 char ch) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = num; // 或者 newNode->data_char = ch;
newNode->next = NULL;
return newNode;
}
// 将节点插入到链表头部
void insert_at_head(Node** head_ref, int num 或者 char ch) {
Node* newNode = create_node(num 或者 ch);
newNode->next = *head_ref;
*head_ref = newNode;
}
int main() {
Node* head = NULL;
printf("请输入数据(0结束):\n");
while (scanf("%d 或 %c", &head->data 或者 &head->data_char) != EOF && head->data != 0 && head->data_char != '\0') { // 检查是否输入了0或空字符
insert_at_head(&head, head->data 或者 head->data_char);
}
// 打印链表
Node* temp = head;
while (temp != NULL) {
printf("%d 或 %c ", temp->data 或者 temp->data_char);
temp = temp->next;
}
printf("\n");
return 0;
}
```
在这个例子中,用户可以连续输入整数或字符直到输入0为止。每次输入都会被转换成对应的链表节点并插入到链表的头部。注意,实际运行过程中,你需要处理错误情况,比如输入不是数字或字符、内存分配失败等情况。
阅读全文
相关推荐
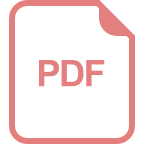
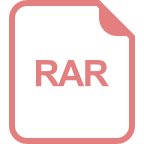
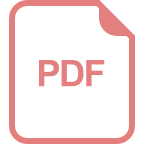
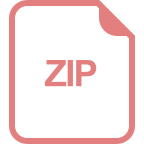
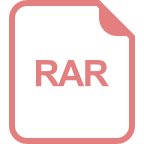
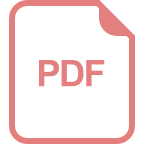
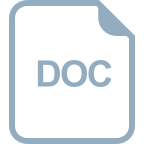
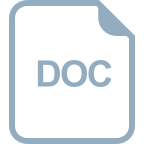
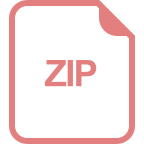
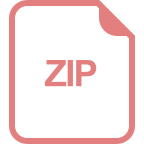
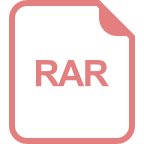
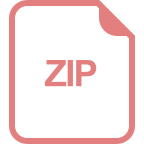
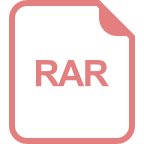
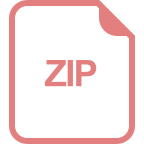