如何使用Python和OpenCV加载并测试一个ONNX格式的人脸检测模型?
时间: 2024-11-01 14:20:39 浏览: 41
要使用Python和OpenCV加载并测试ONNX格式的人脸检测模型,首先需要确保你的开发环境中安装了必要的库。对于Python,你需要安装opencv-python和onnxruntime。可以通过pip安装这些库,如下所示:\n\npip install opencv-python onnxruntime\n\n接下来,你可以使用以下步骤和示例代码来加载和测试你的模型:\n\n1. 导入必要的库:\n\n```python\nimport cv2\nimport numpy as np\nimport onnxruntime\n```\n\n2. 加载ONNX模型:\n\n```python\n# 替换'path_to_onnx_model.onnx'为你的ONNX模型路径\nmodel_path = 'path_to_onnx_model.onnx'\nort_session = onnxruntime.InferenceSession(model_path)\n\n# 获取模型的输入和输出信息\ninput_name = ort_session.get_inputs()[0].name\noutput_name = ort_session.get_outputs()[0].name\n```\n\n3. 读取并预处理图像:\n\n```python\n# 读取图像\nimage_path = 'path_to_image.jpg'\nimg = cv2.imread(image_path)\nimg = cv2.resize(img, (640, 480)) # 调整图像大小以匹配模型输入\nimg = img / 255.0 # 归一化\nimg = img.astype('float32')\nimg = np.expand_dims(img, axis=0) # 增加批次维度\n```\n\n4. 使用模型进行预测:\n\n```python\n# 执行模型推理\nresults = ort_session.run([output_name], {input_name: img})\n```\n\n5. 处理结果:\n\n```python\n# 解析模型输出结果\ndetections = results[0] # 假设输出是一个包含检测结果的Numpy数组\n```\n\n6. 将检测结果绘制在原图上:\n\n```python\n# 假设detections的格式为[x_min, y_min, width, height, confidence]\nfor detection in detections:\n x_min, y_min, width, height, confidence = detection\n if confidence > 0.5: # 置信度阈值可以根据实际情况调整\n # 绘制矩形框和置信度文本\n x_min = int(x_min * img.shape[2])\n y_min = int(y_min * img.shape[1])\n x_max = x_min + int(width * img.shape[2])\n y_max = y_min + int(height * img.shape[1])\n cv2.rectangle(img, (x_min, y_min), (x_max, y_max), (0, 255, 0), 2)\n cv2.putText(img, f'{confidence:.2f}', (x_min, y_min - 5),\n cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)\n\n# 显示结果图像\ncv2.imshow('Face Detection', img)\ncv2.waitKey(0)\n```\n\n通过这些步骤,你可以加载并测试ONNX格式的人脸检测模型,并可视化检测结果。建议深入阅读《使用Python和OpenCV加载ONNX人脸检测模型测试》资源,它将为你提供更深入的理解和更多的实际操作示例。
参考资源链接:[使用Python和OpenCV加载ONNX人脸检测模型测试](https://wenku.csdn.net/doc/5zzbxvnown?spm=1055.2569.3001.10343)
阅读全文
相关推荐
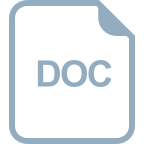
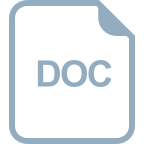
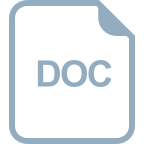




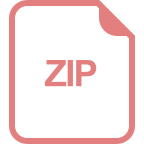
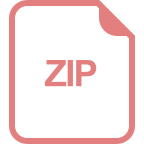
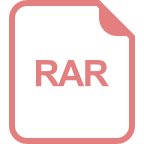
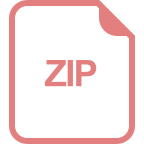
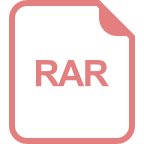
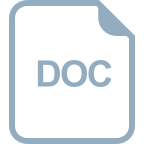



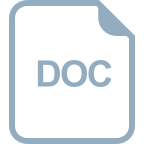
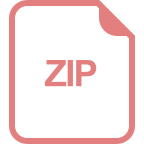