c++ 文件上传下载 pgslq minio
时间: 2024-12-11 20:26:36 浏览: 5
C++文件上传下载到MinIO(一种开源的对象存储服务)通常会利用MinIO SDK提供的API。MinIO SDK为多种编程语言如C++提供了库,方便集成到项目中进行文件操作。
**C++上传文件到MinIO:**
首先,你需要安装MinIO C++客户端库,然后通过以下步骤上传文件:
```cpp
#include <minio/minio.h>
std::string endpoint = "http://your-minio-endpoint:9000"; // MinIO服务器地址
std::string accessKey = "your-access-key";
std::string secretKey = "your-secret-key";
// 创建一个上下文对象
MinioClient minioClient(endpoint, accessKey, secretKey);
// 指定bucket名称和文件路径
std::string bucketName = "my-bucket";
std::string objectName = "path/to/your/file.txt";
try {
PutObjectRequest putObjRequest(bucketName, objectName, "/path/to/local/file.txt"); // 第二个参数是目标文件名
int ret = minioClient.PutObject(putObjRequest);
if (ret >= 0) {
std::cout << "File uploaded successfully." << std::endl;
} else {
std::cerr << "Error uploading file: " << minioClient.GetError() << std::endl;
}
} catch (const MinioException& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
```
**C++下载文件从MinIO:**
下载文件也通过类似的方式,创建请求并执行:
```cpp
GetObjectResult getObjectResult;
GetObjectRequest getRequest(bucketName, objectName);
try {
int ret = minioClient.GetObject(getRequest, &getObjectResult);
if (ret >= 0) {
// 使用文件流保存下载的数据
std::ofstream outputFile("downloaded-file.txt");
outputFile.write(getObjectResult.data(), getObjectResult.size());
outputFile.close();
std::cout << "File downloaded successfully." << std::endl;
} else {
std::cerr << "Error downloading file: " << minioClient.GetError() << std::endl;
}
} catch (const MinioException& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
```
阅读全文
相关推荐
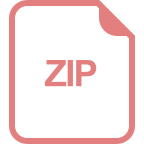
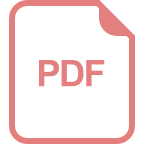
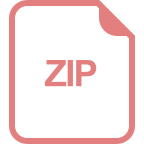















