c++语言用户从键盘上输入姓名和省份证号码,输出姓名,出生年月日及当前的年龄,要求使用C语言库函数#include<ctime.h>
时间: 2024-09-24 18:30:51 浏览: 29
在C++中,为了实现这个功能,你可以按照以下步骤编写程序:
1. 首先,你需要包含必要的头文件`<iostream>`用于标准输入输出操作,以及`<string>`处理字符串,因为姓名通常是字符数组或字符串。尽管题目要求了`<ctime.h>`,但实际上我们并不需要它来获取用户输入和计算年龄,而是使用`<chrono>`库更为合适。
```cpp
#include <iostream>
#include <string>
#include <chrono>
// 定义结构体存储姓名、生日信息
struct PersonalInfo {
std::string name;
int birthYear;
int birthMonth;
int birthDay;
};
int main() {
// 用户输入姓名
std::cout << "请输入您的姓名: ";
std::getline(std::cin, PersonalInfo.name);
// 用户输入出生年月日(假设用户会输入四位数的年份)
std::cout << "请输入您的出生年份(YYYY): ";
std::cin >> PersonalInfo.birthYear;
std::cout << "请输入您的出生月份(MM): ";
std::cin >> PersonalInfo.birthMonth;
std::cout << "请输入您的出生日期/DD: ";
std::cin >> PersonalInfo.birthDay;
// 确保年份是四位数
if (PersonalInfo.birthYear < 100) {
PersonalInfo.birthYear += 2000; // 如果不足四位,则自动加上2000前缀
}
// 当前时间
auto now = std::chrono::system_clock::now();
auto now_time_point = std::chrono::system_clock::to_time_t(now);
time_t current_time = now_time_point;
tm* currentTime = localtime(¤t_time);
// 计算并输出年龄
int currentYear = currentTime->tm_year + 1900;
int age = currentYear - PersonalInfo.birthYear;
if (currentTime->tm_mon > PersonalInfo.birthMonth || (currentTime->tm_mon == PersonalInfo.birthMonth && currentTime->tm_mday > PersonalInfo.birthDay)) {
age--;
}
std::cout << "您的姓名是: " << PersonalInfo.name << "\n";
std::cout << "您的出生日期是: " << PersonalInfo.birthYear << "-" << PersonalInfo.birthMonth << "-" << PersonalInfo.birthDay << "\n";
std::cout << "您的当前年龄是: " << age << "岁" << std::endl;
return 0;
}
```
相关推荐
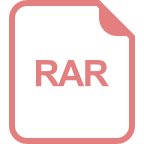
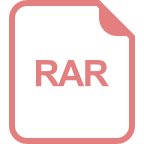
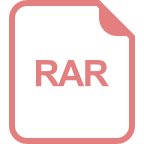














