描述一个圆柱的类。成员中有私有数据半径及高,要求有析构函数、构造函数、体积函数、表面积函数。定义类的1个对象,编写主函数进行测试。C++
时间: 2024-11-23 21:41:10 浏览: 16
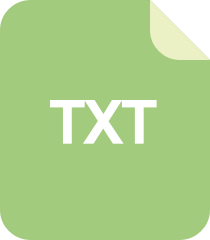
圆柱的表面积及体积(调用函数).txt

在C++中,你可以创建一个名为`Cylinder`的类来描述圆柱体,包含私有变量半径(radius)和高(height),以及相应的公共成员函数(构造函数、析构函数、计算体积和表面积的函数)。下面是一个简单的示例:
```cpp
#include <iostream>
#include <cmath> // 包含数学库用于计算pi
class Cylinder {
private:
double radius; // 半径
double height; // 高度
public:
// 构造函数
Cylinder(double r, double h) : radius(r), height(h) {}
// 析构函数(默认的析构函数通常不需要显式声明)
~Cylinder() {}
// 计算体积的成员函数
double getVolume() const {
return M_PI * pow(radius, 2) * height;
}
// 计算表面积的成员函数
double getSurfaceArea() const {
return 2 * M_PI * radius * (radius + height);
}
// 主函数用于测试
static void testCylinder() {
Cylinder cylinder(5, 10); // 创建一个圆柱对象
std::cout << "Cylinder volume: " << cylinder.getVolume() << std::endl;
std::cout << "Cylinder surface area: " << cylinder.getSurfaceArea() << std::endl;
}
};
int main() {
// 测试圆柱类
Cylinder::testCylinder();
return 0;
}
```
在这个例子中,我们首先定义了一个`Cylinder`类,然后创建了构造函数设置初始值。`getVolume()`和`getSurfaceArea()`函数分别计算并返回体积和表面积。`testCylinder()`函数是用于展示如何使用这个类的一个辅助函数。在`main()`函数里,我们创建了一个`Cylinder`对象,并打印出其体积和表面积。
阅读全文
相关推荐
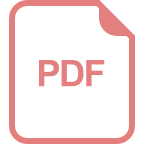
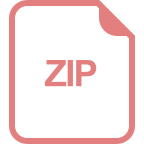

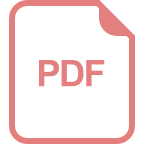
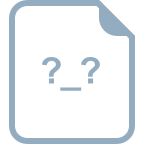
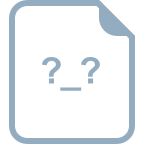
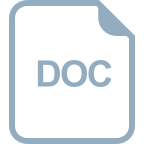
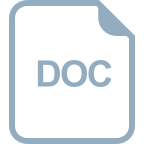
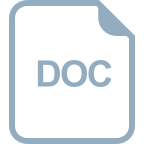
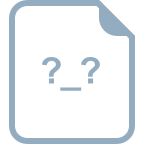
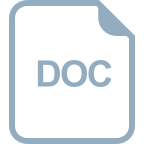
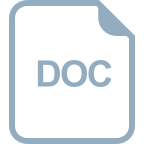
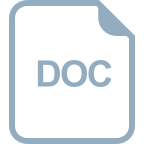
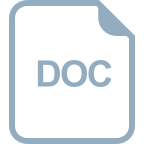
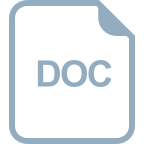
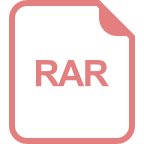
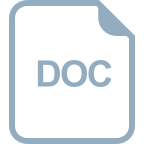
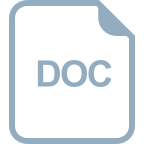