give all code in engllisfh
时间: 2024-10-20 16:12:41 浏览: 16
Sure, I can provide explanations and examples using both Chinese and English. Let me know how you prefer the information.
For a detailed introduction on code structure:
In object-oriented programming (OOP), one key concept is polymorphism, which allows objects of different classes to respond differently to the same method call. Here's a simple explanation with code examples in C++:
**Polymorphism:**
- **Method Overriding**: This is where a subclass provides its own implementation for a method defined in its superclass. The method name must be the same, and it should have the same access level.
```cpp
class Animal {
public:
virtual void makeSound() { cout << "Animal makes sound" << endl; }
};
class Dog : public Animal {
public:
void makeSound() override { cout << "Dog barks" << endl; }
};
int main() {
Animal* animal = new Dog();
animal->makeSound(); // Outputs "Dog barks"
return 0;
}
```
**Virtual Functions:**
- `virtual` keyword before a function declaration indicates that it can be overridden by subclasses.
- A pure virtual function is declared without an implementation but must be implemented in derived classes, marked as `= 0`.
```cpp
class Shape {
public:
virtual ~Shape() {} // Virtual destructor
virtual double area() const = 0; // Pure virtual function
};
class Circle : public Shape {
private:
double radius;
public:
Circle(double r) : radius(r) {}
double area() const override { return M_PI * radius * radius; }
};
```
**Pure Virtual Function:**
- A class containing at least one pure virtual function is abstract, meaning it cannot be instantiated directly.
- Derived classes must implement these functions or also become abstract.
**Virtually Deleted Destructor:**
- Sometimes, a base class needs to ensure a specific behavior for its destructor even if it doesn't manage any resources directly. A virtual destructor is marked as `virtual` and ensures proper cleanup.
```cpp
class Base {
public:
virtual ~Base() {} // Virtual destructor
};
class Derived : public Base {
public:
// No need to define the destructor explicitly
};
```
阅读全文
相关推荐
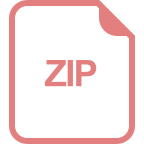
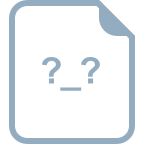
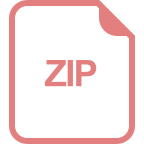
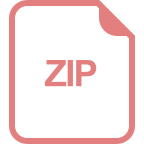
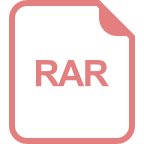
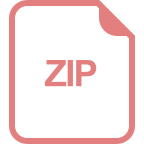
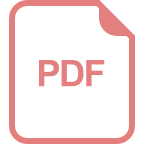
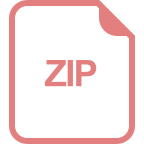
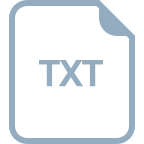
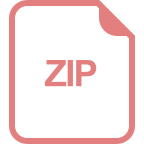
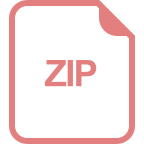
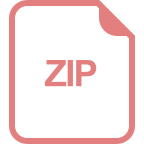
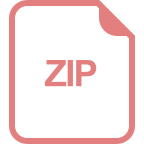
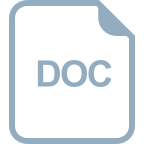
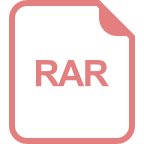
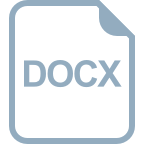
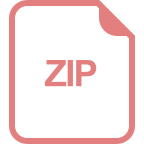