Python给定一个字符串,找到它的第一个不重复字符,并返回它的索引。如果不存在,返回-1
时间: 2024-09-25 18:17:47 浏览: 49
在Python中,可以使用字典数据结构来辅助找到字符串的第一个不重复字符及其索引。以下是一个简单的函数实现:
```python
def first_unique_char(s):
char_count = {} # 创建一个空字典用于存储字符及其计数
for index, char in enumerate(s): # 遍历字符串
if char in char_count:
char_count[char] += 1 # 如果字符已存在,则计数加一
else:
char_count[char] = 1 # 对新字符添加计数
for index, count in char_count.items(): # 再次遍历查找计数为1的字符
if count == 1:
return index # 找到第一个不重复的字符索引,立即返回
return -1 # 如果找不到不重复的字符,返回-1
# 示例
s = "leetcode"
print(first_unique_char(s)) # 输出:0,因为'l'是第一个不重复字符,索引为0
```
这个函数首先统计每个字符的出现次数,然后再次遍历字典找出第一个出现次数为1的字符,即为第一个不重复字符。
相关问题
Python给定一个字符串,找到它的第一个不重复的字符,并返回它的索引。如果不存在,则返回 -1。
给定一个字符串,找到它的第一个不重复的字符,并返回它的索引。如果不存在,则返回 -1。
方法一:
```python
def first_not_repeating_char(strings):
chars = []
times = []
for s in strings:
if s not in chars:
chars.append(s)
times.append(1)
else:
char_index = chars.index(s)
times[char_index] += 1
for t in times:
if t == 1:
times_index = times.index(t)
return chars[times_index]
return -1
strings = input('请输入长字符串:')
print('结果:', first_not_repeating_char(strings))
```
方法二:
```python
from collections import Counter
def deal_str(s):
counter = Counter(s)
singles = [_c for _c, _i in counter.items() if _i == 1]
idxs = [s.index(_c) for _c in singles]
idx = min(idxs) if idxs else -1
print(f"字符串({s})中的第一个不重复字符的位置是({idx})")
string = "abcab"
deal_str(string)
```
方法三:
```python
def deal_str2(s):
my_dict = {}
idx = -1
for _i, _c in enumerate(s):
if _c not in my_dict:
my_dict[_c] = _i
else:
my_dict[_c] = 9999
idx = min(my_dict.values())
idx = -1 if idx >= 9999 else idx
print(f"字符串({s})中的第一个不重复字符的位置是({idx})")
string = "abcab"
deal_str2(string)
```
python获取字符串中的第一个唯一字符。 要求:给定一个字符串,找到它的第一个不重复的字符,并返回它的索引。如果不存在,则返回 -1。
在Python中,你可以通过创建一个字典来跟踪每个字符的出现次数,然后遍历字符串查找第一个未出现在字典中的字符。以下是一个简单的函数实现这个功能:
```python
def first_unique_char(s):
char_count = {}
for i, char in enumerate(s):
if char in char_count:
char_count[char] += 1
else:
char_count[char] = 1
for index, char in enumerate(s):
if char_count[char] == 1:
return index
# 如果没有找到唯一的字符,返回 -1
return -1
```
在这个函数中,我们首先初始化一个空字典`char_count`,然后遍历输入字符串`s`的每一个字符。对于每个字符,如果它已经在字典中,我们就增加计数;如果不在,就添加到字典并设置计数为1。接着,我们再次遍历字符串,当遇到计数为1的字符时,说明找到了第一个唯一字符,返回其索引。如果没有找到这样的字符,函数最后返回-1。
阅读全文
相关推荐
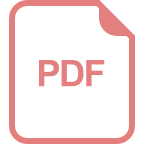
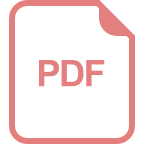
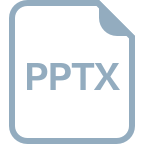













