golang绘图如何让freetype绘制的文字水平居中
时间: 2024-09-21 07:03:10 浏览: 51
在 Go 语言中,要使用 Freetype 来绘制文本并使其水平居中,你可以利用 `github.com/golang/freetype/truetype` 包提供的功能,结合图像处理库如 `image` 和 `draw`。以下是一个简单的步骤:
1. 首先,你需要加载字体文件到一个 `truetype.Font` 对象:
```go
fontBytes, err := ioutil.ReadFile("your_font.ttf") // 替换为你的字体文件路径
if err != nil {
// 处理错误
}
fontFace, err := ttf.Parse(fontBytes)
if err != nil {
// 处理错误
}
```
2. 创建一个画布,并设置尺寸,以便容纳你要绘制的文本:
```go
imgWidth := 500 // 你想让图片的宽度是多少
imgHeight := int(math.Max(float64(fontFace.UnitsPerEm) * len(yourText), yourDesiredHeight)) // 根据文本长度计算高度
img := image.NewRGBA(image.Rect(0, 0, imgWidth, imgHeight))
```
3. 使用 `DrawString` 函数从字体 Face 中创建一个渲染上下文,并指定文字、颜色以及坐标(这里我们假设你想要水平居中,所以 x 应该是宽度的一半减去文字宽度的一半):
```go
ctx := freetype.NewContext()
ctx.SetFontFace(fontFace)
ctx.SetFontSize(fontSize)
x := (imgWidth - ctx.PointToPxAdvance([]rune(yourText)[0])) / 2
y := imgHeight - ctx.Size.Y
_, baseline := ctx.DrawString(yourText, x, y)
```
4. 最后,将绘制的内容复制到图像上:
```go
draw.Draw(img, img.Bounds(), &image.Uniform{color.White}, image.ZP, draw.Src)
// 绘制黑色文字
draw.Draw(img, img.Bounds().Add(image.Pt(x, y-baseline)), blackImage, image.ZP, draw.Src)
```
这里的 `blackImage` 是一个已经填充黑色的图像。
阅读全文
相关推荐
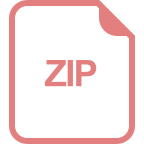
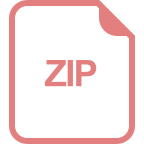
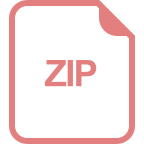

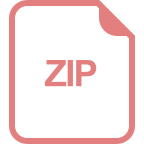
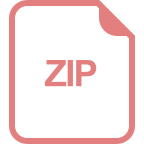
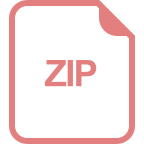
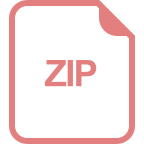
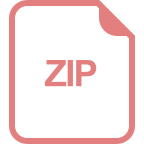
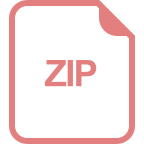
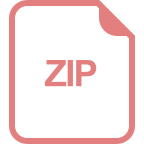
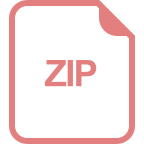
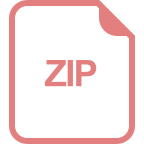