前端获取get请求后的headers中的patientID
时间: 2024-09-30 18:01:51 浏览: 27
前端通过JavaScript可以访问HTTP响应头,包括`PatientID`等信息。通常在处理GET请求后,你可以使用Fetch API、XMLHttpRequest(XHR)或者其他库(如axios)来获取数据和响应头。这里是一个简单的例子,假设你使用的是fetch:
```javascript
fetch('your-api-url')
.then(response => response.headers)
.then(headers => headers.get('PatientID'))
.then(patientID => {
// 使用获取到的patientID
console.log('Patient ID:', patientID);
})
.catch(error => {
// 处理错误
console.error('Error getting PatientID:', error);
});
```
在这个代码片段中,首先发送一个GET请求,然后从返回的response对象中获取headers,再通过`.get()`方法获取特定的header值(这里是`PatientID`)。如果成功获取到,它会被打印出来;如果有错误,会捕获并记录。
相关问题
前端获取当前网页的headers中的patientID
在前端JavaScript中,你可以使用`XMLHttpRequest`对象或者更现代的`fetch` API 来获取HTTP响应头。如果你想要获取当前网页的headers中的patientID,通常这个信息不会直接包含在响应头中,因为headers是服务器与客户端通信的一部分,而patientID通常是服务器返回的数据的一部分。
如果你想获取某个特定HTTP头(比如通过cookie传递的patientID),可以这样做:
```javascript
// XMLHttpRequest示例
var xhr = new XMLHttpRequest();
xhr.open('GET', '/', true);
xhr.onreadystatechange = function () {
if (xhr.readyState === XMLHttpRequest.DONE && xhr.status === 200) {
var headers = xhr.getAllResponseHeaders(); // 获取所有响应头
console.log(headers); // 查找"Set-Cookie"部分,其中可能包含了patientID
// 或者从cookies中解析,例如:
var cookies = xhr.getResponseHeader('Set-Cookie');
if (cookies) {
var cookieArray = cookies.split('; ');
for (var i = 0; i < cookieArray.length; i++) {
var cookiePair = cookieArray[i].split('=');
if (cookiePair[0] === 'patientID') { // 检查是否为patientID
console.log(cookiePair[1]); // 获取patientID值
}
}
}
}
};
xhr.send();
// fetch 示例(异步)
fetch('/')
.then(response => response.headers)
.then(headers => {
console.log(headers.get('Set-Cookie')); // 类似于上面的检查
});
```
注意,这通常需要服务器设置正确的`Access-Control-Allow-Origin`头部以允许跨域请求,并且某些敏感信息如patientID可能会经过加密或隐藏在URL查询参数、POST数据或session storage中,而不是直接在headers里。
前端获取当前网页已存在的headers中的patientID
前端JavaScript通过`document`对象的`fetch` API 或者 `XMLHttpRequest` 可以获取到当前网页中的HTTP响应头信息,包括`patientID`。这里是一个简单的示例:
```javascript
// 使用 Fetch API
async function getPatientID() {
const response = await fetch(window.location.href);
if (response.ok) {
const headers = response.headers;
let patientID = headers.get('patientID'); // 获取名为 'patientID' 的header值
console.log(patientID); // 打印 patientID
} else {
console.error('Failed to fetch patient ID', response.status);
}
}
// 使用 XMLHttpRequest
function getPatientIDOldSchool() {
const xhr = new XMLHttpRequest();
xhr.open('GET', window.location.href, true);
xhr.onreadystatechange = function () {
if (xhr.readyState === XMLHttpRequest.DONE && xhr.status === 200) {
const headers = xhr.getAllResponseHeaders().split('\n');
for (let header of headers) {
if (header.includes('Content-Type')) { // 这里假设 patientID 存在于 Content-Type 中
const [name, value] = header.split(': ');
if (name.toLowerCase() === 'patient-id') { // 更改名称检查,如果原名不是 'patientID'
console.log(value);
break; // 找到后就停止循环
}
}
}
}
};
xhr.send();
}
// 调用其中一个函数来获取
getPatientID(); // 或 getPatientIDOldSchool();
```
请注意,实际的`patientID`字段可能会出现在不同的HTTP头名称中,这取决于服务器设置。上述代码仅展示了基本的查找过程。
阅读全文
相关推荐
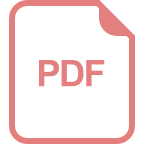
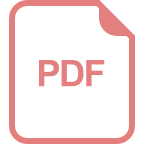
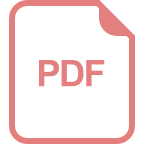

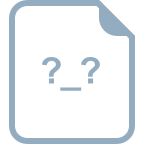
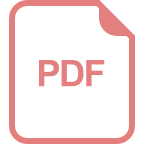
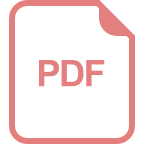
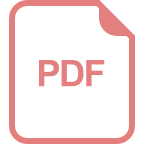
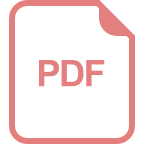
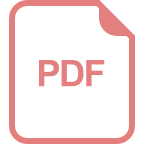
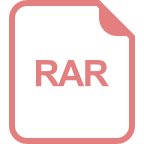
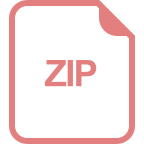
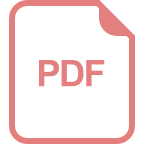
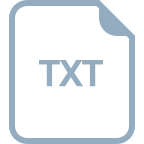
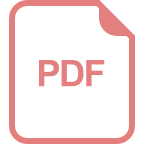