基于java的药店库存管理系统的设计与实现的代码
时间: 2024-09-15 22:02:36 浏览: 43
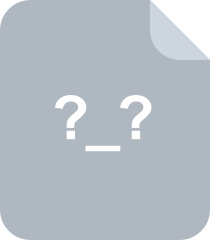
基于SSM框架的库存管理系统的设计与实现

基于 Java 的药店库存管理系统通常会采用分层架构设计,主要包括业务逻辑层、数据访问层和用户界面层。以下是一个简化的概述:
1. **业务逻辑层 (Business Logic Layer)**:
- **实体类**:如`Drug`, `StockRecord`, 等,表示药品、库存记录等实体。
- **服务类**:如`InventoryService`, 提供添加药品、更新库存、查询库存操作的方法。
```java
public class InventoryService {
private DrugRepository drugRepository;
public InventoryService(DrugRepository repository) {
this.drugRepository = repository;
}
// 添加药品
public void addDrug(Drug drug) {
drugRepository.save(drug);
}
// 更新库存
public void updateStock(StockRecord record) {
drugRepository.update(record);
}
// 查询库存
public StockRecord getStockById(String药ID) {
return drugRepository.findById(药ID).orElse(null);
}
}
```
2. **数据访问层 (Data Access Layer)**:
- **仓库接口**:`DrugRepository`,定义了CRUD操作,如`save()`, `findById()`, `update()` 等。
- **具体实现**:可以使用JPA(Java Persistence API)或者MyBatis等框架,通过数据库操作库存数据。
```java
@Repository
public interface DrugRepository extends JpaRepository<Drug, Long> {
Optional<StockRecord> findById(String id);
// 其他 CRUD 方法...
}
```
3. **用户界面层 (User Interface Layer)**:
- **控制器**:如`InventoryController`, 接受前端请求并调用业务逻辑层的服务方法。
- **前端**:使用HTML/CSS/JavaScript构建界面,展示库存信息和交互功能。
```java
@Controller
@RequestMapping("/inventory")
public class InventoryController {
@Autowired
private InventoryService inventoryService;
@GetMapping("/{drugId}")
public String viewStock(@PathVariable String drugId) {
StockRecord stock = inventoryService.getStockById(drugId);
return "inventory", stock;
}
// 其他处理方法...
}
```
阅读全文
相关推荐
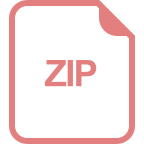
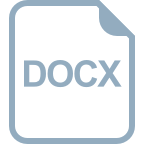















