C#脚本 一个单位从路径1走到路径6
时间: 2024-10-25 21:13:24 浏览: 10
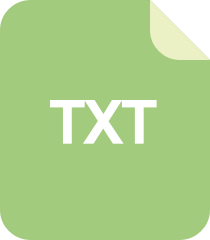
AE+C# 最短路径

在C#中,如果需要编写一个脚本来控制游戏中的角色从路径1移动到路径6,通常会涉及以下几个步骤:
1. **初始化**:首先,你需要创建一个表示单位的类,包含位置信息以及表示当前路径的状态。
```csharp
public class Unit {
public Vector3 CurrentPosition; // 单位当前位置
public int CurrentPathIndex; // 当前路径索引,从1开始
// 添加路径数据字段和属性...
}
```
2. **定义路径**:为每个路径(如路径1到路径6)创建一个路径点数组,或者使用Vector3[]或其他适合的数据结构存储路径节点。
```csharp
List<Vector3> Path1 = ...;
List<Vector3> Path6 = ...;
```
3. **移动函数**:编写一个方法来计算并更新单位的位置,使其沿着当前路径移动。
```csharp
void MoveTowardsNextWaypoint(Unit unit) {
if (unit.CurrentPathIndex < Path6.Count) {
Vector3 nextWaypoint = Path6[unit.CurrentPathIndex];
float distanceToReach = Vector3.Distance(unit.CurrentPosition, nextWaypoint);
// 使用平滑运动算法(如EaseInOut等)
unit.CurrentPosition += (nextWaypoint - unit.CurrentPosition) * Time.deltaTime * MovementSpeed;
// 到达目标点
if (distanceToReach <= MovementThreshold) {
unit.CurrentPathIndex++;
}
} else {
// 如果到达了路径终点,可以切换到下一个路径,或者完成任务
// ...
}
}
```
4. **定时更新**:在游戏循环中定期调用`MoveTowardsNextWaypoint`函数来更新单位位置。
5. **处理状态转换**:当单位从一个路径切换到另一个路径时,需要改变`CurrentPathIndex`和相关的路径节点。
```csharp
void ChangePath(int newPathIndex) {
unit.CurrentPathIndex = newPathIndex;
unit.CurrentPosition = Path[newPathIndex][0]; // 设置新路径的第一个节点作为起始位置
}
```
阅读全文
相关推荐
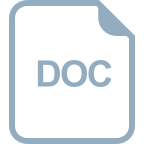
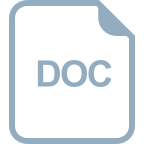
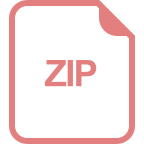
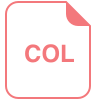
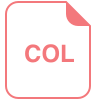
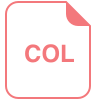
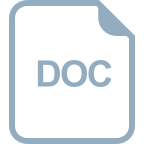
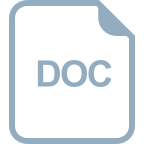
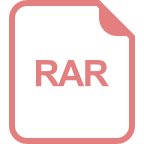
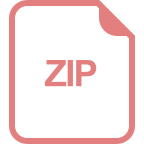