java web程序实现接收iPhone动态图
时间: 2024-12-21 20:06:08 浏览: 4
在Java Web程序中实现接收iPhone同步图(如Live Photo)通常涉及以下几个步骤:
1. **前端上传动态图**:
- 使用HTML和JavaScript创建一个上传表单,允许用户选择并上传动态图。
- 由于Live Photo包含一个视频文件和一张静态图片文件,可以通过JavaScript将这两个文件一起上传。
2. **后端接收和处理文件**:
- 使用Spring Boot或其他Java Web框架创建一个后端服务,接收上传的文件。
- 后端需要处理多个文件(视频和图片),并将其保存到服务器上的指定目录。
3. **存储和管理动态图**:
- 将上传的动态图文件存储在服务器的文件系统中,或者存储在云存储服务中。
- 使用数据库记录每个动态图的相关信息,如文件路径、上传时间、用户信息等。
以下是一个简单的示例代码,展示如何在Spring Boot中实现接收iPhone动态图:
### 前端HTML表单
```html
<!DOCTYPE html>
<html>
<head>
<title>上传动态图</title>
</head>
<body>
<h2>上传iPhone动态图</h2>
<form action="/upload" method="post" enctype="multipart/form-data">
<input type="file" name="livePhoto" accept="image/*,video/*" multiple>
<input type="submit" value="上传">
</form>
</body>
</html>
```
### 后端Spring Boot代码
```java
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
import org.springframework.web.bind.annotation.RequestMapping;
import java.io.File;
import java.io.IOException;
@RestController
public class LivePhotoController {
@PostMapping("/upload")
public String handleFileUpload(@RequestParam("livePhoto") MultipartFile[] files) {
String uploadDir = "uploads/";
File dir = new File(uploadDir);
if (!dir.exists()) {
dir.mkdir();
}
for (MultipartFile file : files) {
if (file.isEmpty()) {
return "文件为空";
}
try {
String fileName = file.getOriginalFilename();
File serverFile = new File(uploadDir + fileName);
file.transferTo(serverFile);
} catch (IOException e) {
return "文件上传失败: " + e.getMessage();
}
}
return "文件上传成功";
}
}
```
### 数据库记录(示例)
```sql
CREATE TABLE live_photos (
id INT AUTO_INCREMENT PRIMARY KEY,
file_path VARCHAR(255) NOT NULL,
upload_time TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
```
### 数据库操作(示例)
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.stereotype.Repository;
@Repository
public class LivePhotoRepository {
@Autowired
private JdbcTemplate jdbcTemplate;
public void saveFilePath(String filePath) {
String sql = "INSERT INTO live_photos (file_path) VALUES (?)";
jdbcTemplate.update(sql, filePath);
}
}
```
### 后端完整代码
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
import org.springframework.web.bind.annotation.RequestMapping;
import java.io.File;
import java.io.IOException;
@RestController
public class LivePhotoController {
@Autowired
private LivePhotoRepository livePhotoRepository;
@PostMapping("/upload")
public String handleFileUpload(@RequestParam("livePhoto") MultipartFile[] files) {
String uploadDir = "uploads/";
File dir = new File(uploadDir);
if (!dir.exists()) {
dir.mkdir();
}
for (MultipartFile file : files) {
if (file.isEmpty()) {
return "文件为空";
}
try {
String fileName = file.getOriginalFilename();
File serverFile = new File(uploadDir + fileName);
file.transferTo(serverFile);
livePhotoRepository.saveFilePath(serverFile.getAbsolutePath());
} catch (IOException e) {
return "文件上传失败: " + e.getMessage();
}
}
return "文件上传成功";
}
}
```
阅读全文
相关推荐
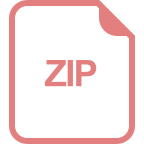
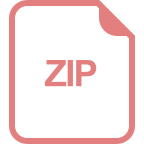
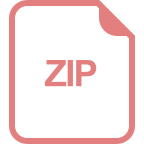
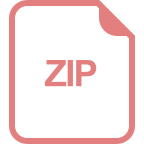
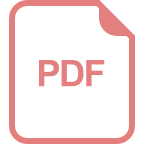
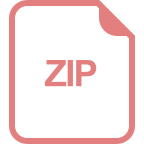
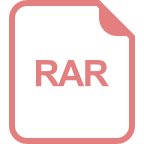
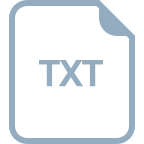
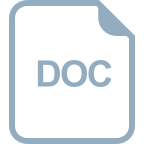
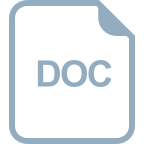
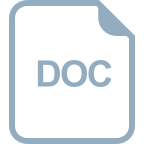
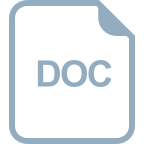
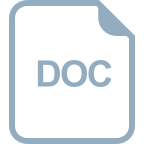
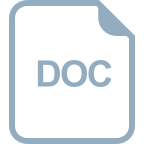
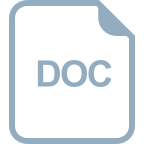
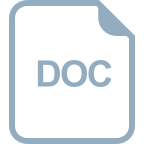
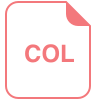
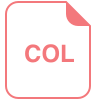