javamail发送邮件设置
时间: 2024-05-17 17:16:20 浏览: 19
要设置JavaMail发送邮件,你需要按照以下步骤进行操作:
1. 导入相关的类库:首先,确保你的项目中已经导入了JavaMail和Java Activation Framework(JAF)的相关类库。你可以从官方网站(https://javaee.github.io/javamail/)下载并添加这些类库到你的项目中。
2. 配置SMTP服务器信息:你需要指定SMTP服务器的地址和端口号。通常,SMTP服务器的地址是根据你使用的邮件服务提供商而不同。例如,对于Gmail来说,SMTP服务器地址是"smtp.gmail.com",端口号是587。
3. 创建Session对象:使用javax.mail.Session类创建一个Session对象。你可以通过使用Properties对象来设置Session的一些属性,例如SMTP服务器信息、是否需要身份验证等。
```java
Properties properties = new Properties();
properties.put("mail.smtp.host", "smtp.gmail.com");
properties.put("mail.smtp.port", "587");
properties.put("mail.smtp.auth", "true");
Session session = Session.getDefaultInstance(properties, new javax.mail.Authenticator() {
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication("your-email@gmail.com", "your-password");
}
});
```
在这里,你需要替换`your-email@gmail.com`和`your-password`为你自己的邮箱地址和密码。
4. 创建Message对象:使用javax.mail.Message类创建一个Message对象,并设置邮件的发送者、接收者、主题和正文等信息。
```java
Message message = new MimeMessage(session);
message.setFrom(new InternetAddress("your-email@gmail.com"));
message.setRecipients(Message.RecipientType.TO, InternetAddress.parse("recipient-email@example.com"));
message.setSubject("Hello, World!");
message.setText("This is the content of the email.");
```
在这里,你需要将`your-email@gmail.com`替换为你自己的邮箱地址,将`recipient-email@example.com`替换为邮件接收者的邮箱地址。
5. 发送邮件:使用Transport类的静态方法send()发送邮件。
```java
Transport.send(message);
```
完整的示例代码如下:
```java
import javax.mail.*;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeMessage;
import java.util.Properties;
public class EmailSender {
public static void main(String[] args) {
Properties properties = new Properties();
properties.put("mail.smtp.host", "smtp.gmail.com");
properties.put("mail.smtp.port", "587");
properties.put("mail.smtp.auth", "true");
Session session = Session.getDefaultInstance(properties, new javax.mail.Authenticator() {
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication("your-email@gmail.com", "your-password");
}
});
try {
Message message = new MimeMessage(session);
message.setFrom(new InternetAddress("your-email@gmail.com"));
message.setRecipients(Message.RecipientType.TO, InternetAddress.parse("recipient-email@example.com"));
message.setSubject("Hello, World!");
message.setText("This is the content of the email.");
相关推荐
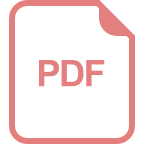
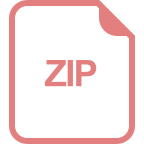














