用随机产生的10个英文字符,分别用rinsert和hinsert,建立单链表,输出结果 c语言
时间: 2024-10-14 16:13:27 浏览: 38
在C语言中,我们可以使用结构体来表示链表节点,并利用`rand()`函数生成随机英文字符。这里我将简要地展示如何创建两个插入操作的函数:`rinsert`(随机插入)和`hinsert`(按字母顺序插入),然后输出链表。
首先,定义链表节点结构:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <string.h>
typedef struct Node {
char data;
struct Node* next;
} Node;
Node* createList(char* str) {
int len = strlen(str);
Node* head = (Node*)malloc(sizeof(Node));
head->data = *str++;
head->next = NULL;
for (int i = 1; i < len; ++i) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = *str++;
newNode->next = head->next;
head->next = newNode;
}
return head;
}
void rinsert(Node** head, Node** tail) {
srand(time(0)); // 初始化随机数种子
char random_char = 'a' + rand() % ('z' - 'a' + 1); // 随机英文字母
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = random_char;
newNode->next = NULL;
if (*tail == NULL) {
*head = newNode;
*tail = newNode;
} else {
newNode->next = *tail;
*tail = newNode;
}
}
void hinsert(Node** head, Node** tail, char key) {
Node* current = *head;
while (current != NULL && current->data < key) {
current = current->next;
}
if (current == NULL) {
newNode = (Node*)malloc(sizeof(Node));
newNode->data = key;
newNode->next = *head;
*head = newNode;
} else {
newNode = (Node*)malloc(sizeof(Node));
newNode->data = key;
newNode->next = current->next;
current->next = newNode;
}
}
void printList(Node* head) {
Node* temp = head;
while (temp != NULL) {
printf("%c -> ", temp->data);
temp = temp->next;
}
printf("NULL\n");
}
int main() {
char random_str[11] = "RandomString"; // 生成随机字符串示例
Node* list = createList(random_str);
Node* rtail = NULL;
for (int i = 0; i < 5; i++) {
rinsert(&list, &rtail);
}
printf("Random Insertion List:\n");
printList(list);
char hkey = random_str[strlen(random_str) / 2]; // 选择一个中间字符作为排序依据
hinsert(&list, &rtail, hkey);
printf("\nAlphabetically Sorted List with Key '%c':\n", hkey);
printList(list);
return 0;
}
```
这个程序首先创建一个包含随机字符的链表,然后用`rinsert`随机插入5个字符,接着使用`hinsert`按照字符顺序插入一个字符并打印最终链表。
阅读全文
相关推荐
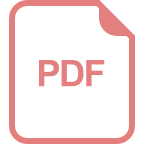


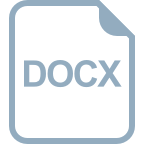
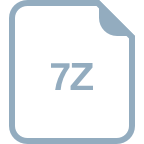
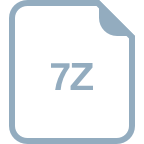
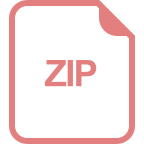
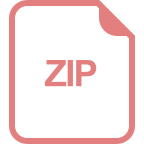
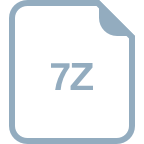
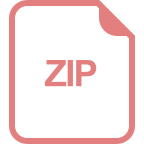
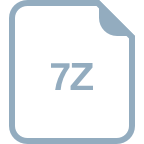
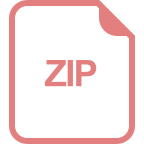
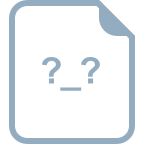