线性表的插入与删除的Dev c++代码
时间: 2024-09-29 10:07:16 浏览: 19
线性表的插入和删除操作在Dev C++中通常涉及数组或动态链表实现。这里是一个简单的单向动态链表(Node结构和基本操作的示例):
```cpp
#include <iostream>
// 定义链表节点
struct Node {
int data;
Node* next;
};
// 创建一个新的链表节点
Node* createNode(int value) {
Node* newNode = new Node();
if (newNode == nullptr) {
std::cout << "Memory allocation failed.\n";
return nullptr;
}
newNode->data = value;
newNode->next = nullptr;
return newNode;
}
// 在链表末尾插入新节点
void insertAtEnd(Node** head, int value) {
if (*head == nullptr) {
*head = createNode(value);
} else {
Node* current = *head;
while (current->next != nullptr) {
current = current->next;
}
current->next = createNode(value);
}
}
// 删除指定值的第一个节点
void deleteNode(Node** head, int value) {
if (*head == nullptr) {
std::cout << "List is empty.\n";
return;
}
if ((*head)->data == value) {
Node* temp = *head;
*head = (*head)->next;
delete temp;
return;
}
Node* current = *head;
while (current->next != nullptr && current->next->data != value) {
current = current->next;
}
if (current->next != nullptr) {
Node* temp = current->next;
current->next = current->next->next;
delete temp;
} else {
std::cout << "Value not found in the list.\n";
}
}
// 打印链表
void printList(Node* node) {
while (node != nullptr) {
std::cout << node->data << " -> ";
node = node->next;
}
std::cout << "nullptr\n";
}
int main() {
Node* head = nullptr;
// 插入操作示例
insertAtEnd(&head, 10);
insertAtEnd(&head, 20);
insertAtEnd(&head, 30);
// 打印初始链表
printList(head);
// 删除操作示例
deleteNode(&head, 20);
// 再次打印链表
printList(head);
return 0;
}
```
在这个例子中,我们首先创建了一个链表,并展示了如何在链表末尾插入节点和删除具有特定值的节点。`main`函数展示了完整的操作流程。
相关推荐
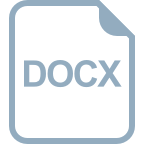
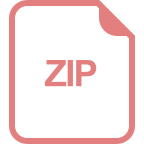
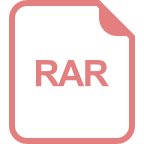







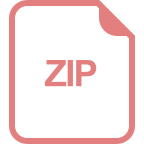
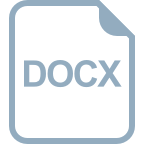
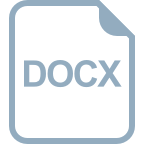