基于dubbo的代码实例
时间: 2024-04-25 10:19:24 浏览: 141
基于dubbo的代码实例可以通过以下步骤来实现[^2]:
1. 首先,确保你已经安装了Zookeeper,并启动了Zookeeper服务。
2. 创建一个Maven项目,并在pom.xml文件中添加Dubbo和Zookeeper的依赖。
3. 创建一个接口,定义需要暴露的服务方法。
```java
public interface HelloService {
String sayHello(String name);
}
```
4. 创建一个实现类,实现接口中定义的服务方法。
```java
public class HelloServiceImpl implements HelloService {
@Override
public String sayHello(String name) {
return "Hello, " + name + "!";
}
}
```
5. 在resources目录下创建一个dubbo配置文件dubbo-provider.xml,配置Dubbo的服务提供者。
```xml
<?xml version="1.0" encoding="UTF-8"?>
<dubbo:application name="dubbo-demo-provider" />
<dubbo:registry address="zookeeper://127.0.0.1:2181" />
<dubbo:protocol name="dubbo" port="20880" />
<dubbo:service interface="com.example.HelloService" ref="helloService" />
<bean id="helloService" class="com.example.HelloServiceImpl" />
```
6. 创建一个启动类,用于启动Dubbo服务提供者。
```java
public class Provider {
public static void main(String[] args) throws IOException {
ClassPathXmlApplicationContext context = new ClassPathXmlApplicationContext("dubbo-provider.xml");
context.start();
System.in.read();
}
}
```
7. 创建一个消费者项目,重复步骤2和3,创建一个接口和实现类。
8. 在resources目录下创建一个dubbo配置文件dubbo-consumer.xml,配置Dubbo的服务消费者。
```xml
<?xml version="1.0" encoding="UTF-8"?>
<dubbo:application name="dubbo-demo-consumer" />
<dubbo:registry address="zookeeper://127.0.0.1:2181" />
<dubbo:reference id="helloService" interface="com.example.HelloService" />
```
9. 创建一个启动类,用于启动Dubbo服务消费者。
```java
public class Consumer {
public static void main(String[] args) {
ClassPathXmlApplicationContext context = new ClassPathXmlApplicationContext("dubbo-consumer.xml");
HelloService helloService = (HelloService) context.getBean("helloService");
String result = helloService.sayHello("World");
System.out.println(result);
}
}
```
以上是一个基于Dubbo的简单代码实例,通过配置Dubbo的服务提供者和消费者,可以实现分布式的服务调用。你可以根据自己的需求进行扩展和定制。如果你想了解更多关于Dubbo的使用和原理,可以参考Dubbo的官方文档和源码[^1]。
阅读全文
相关推荐
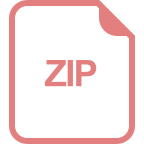
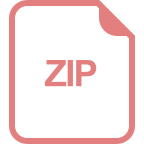
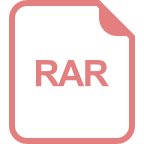
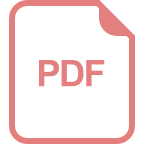
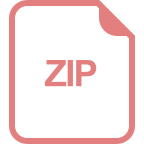
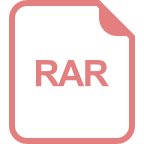
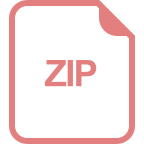
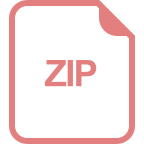
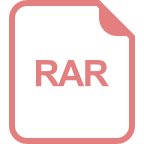
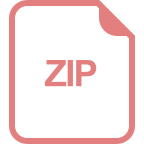
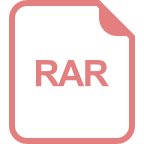
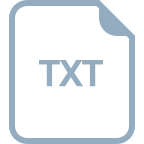
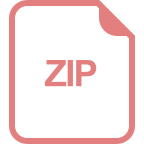
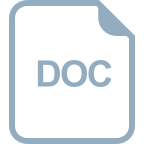