遍历ConcurrentHashMap
时间: 2023-11-15 19:01:53 浏览: 42
遍历ConcurrentHashMap可以使用迭代器,通过调用ConcurrentHashMap的keySet()方法获取key的Set集合,然后使用Set集合的迭代器进行遍历。需要注意的是,由于ConcurrentHashMap是线程安全的,因此在遍历时可能会有其他线程对ConcurrentHashMap进行修改,为了避免ConcurrentModificationException异常,可以使用ConcurrentHashMap的迭代器进行遍历。另外,由于ConcurrentHashMap是无序的,因此遍历时输出的顺序可能是乱序的。
相关问题
遍历concurrenthashmap
在Java中,遍历ConcurrentHashMap有以下几种方式:
1.使用ConcurrentHashMap的keySet()方法获取key的集合,然后遍历key集合,通过get()方法获取对应的value值。
```java
ConcurrentHashMap<String, Integer> map = new ConcurrentHashMap<>();
// 添加元素
Set<String> keySet = map.keySet();
for (String key : keySet) {
Integer value = map.get(key);
System.out.println(key + " : " + value);
}
```
2.使用ConcurrentHashMap的entrySet()方法获取键值对的集合,然后遍历键值对集合,通过getKey()和getValue()方法获取对应的key和value值。
```java
ConcurrentHashMap<String, Integer> map = new ConcurrentHashMap<>();
// 添加元素
Set<Map.Entry<String, Integer>> entrySet = map.entrySet();
for (Map.Entry<String, Integer> entry : entrySet) {
String key = entry.getKey();
Integer value = entry.getValue();
System.out.println(key + " : " + value);
}
```
3.使用ConcurrentHashMap的forEach()方法遍历键值对集合,通过lambda表达式获取对应的key和value值。
```java
ConcurrentHashMap<String, Integer> map = new ConcurrentHashMap<>();
// 添加元素
map.forEach((key, value) -> {
System.out.println(key + " : " + value);
});
```
4.使用ConcurrentHashMap的iterator()方法获取迭代器,然后遍历键值对集合,通过next()方法获取对应的key和value值。
```java
ConcurrentHashMap<String, Integer> map = new ConcurrentHashMap<>();
// 添加元素
Iterator<Map.Entry<String, Integer>> iterator = map.entrySet().iterator();
while (iterator.hasNext()) {
Map.Entry<String, Integer> entry = iterator.next();
String key = entry.getKey();
Integer value = entry.getValue();
System.out.println(key + " : " + value);
}
```
java遍历ConcurrentHashMap
Java中的ConcurrentHashMap是一个线程安全的哈希表,它支持高并发,而且可以在多线程的情况下保证数据的一致性。遍历ConcurrentHashMap有以下两种方法:
1.使用迭代器Iterator遍历
可以通过ConcurrentHashMap的keySet()、entrySet()、values()方法获取迭代器,然后使用迭代器遍历ConcurrentHashMap。
```
ConcurrentHashMap<String, Integer> map = new ConcurrentHashMap<>();
//添加元素
map.put("A", 1);
map.put("B", 2);
map.put("C", 3);
//使用迭代器遍历
Iterator<Map.Entry<String, Integer>> iterator = map.entrySet().iterator();
while(iterator.hasNext()){
Map.Entry<String, Integer> entry = iterator.next();
System.out.println(entry.getKey() + ": " + entry.getValue());
}
```
2.使用forEach遍历
Java 8引入了forEach方法,可以使用lambda表达式遍历ConcurrentHashMap。
```
ConcurrentHashMap<String, Integer> map = new ConcurrentHashMap<>();
//添加元素
map.put("A", 1);
map.put("B", 2);
map.put("C", 3);
//使用forEach遍历
map.forEach((key, value) -> System.out.println(key + ": " + value));
```
相关推荐
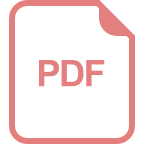
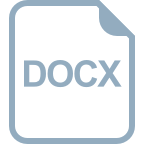
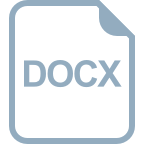












