发udp数据包和收udp数据包可以在一个Python代码中完成吗?
时间: 2024-09-26 21:13:03 浏览: 37
当然可以,在Python中,你可以使用内置的socket模块来发送和接收UDP(用户数据报协议)数据包。以下是一个简单的例子:
```python
import socket
def send_udp_packet(host, port, message):
# 创建一个UDP套接字
sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
# 组织数据包的内容(包括消息和端口号)
data = (message, port)
try:
# 发送数据到指定主机和端口
sock.sendto(data, (host, port))
print(f"Sent: {message} to {host}:{port}")
except Exception as e:
print(f"Error sending UDP packet: {str(e)}")
finally:
# 关闭套接字
sock.close()
def receive_udp_packet(port):
# 创建一个UDP套接字,用于接收
sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM, socket.IPPROTO_UDP)
sock.bind(('0.0.0.0', port)) # 使用localhost地址以便监听所有接口
try:
while True:
# 接收并打印收到的数据
message, address = sock.recvfrom(4096) # 数据大小限制
print(f"Received: {message.decode()} from {address[0]}:{address[1]}")
except KeyboardInterrupt:
print("Closing the server...")
finally:
# 关闭套接字
sock.close()
# 调用函数发送和接收数据
send_udp_packet('target_host', target_port, 'Hello, UDP!')
receive_udp_packet(receive_port)
```
在这个例子中,`send_udp_packet`函数负责发送数据,而`receive_udp_packet`函数监听指定端口等待接收数据。你可以根据需要调整参数并设置两个函数分别运行。
阅读全文
相关推荐
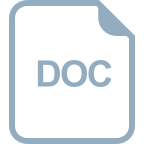
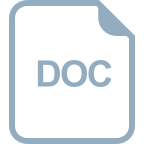
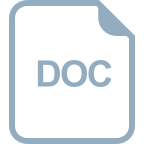

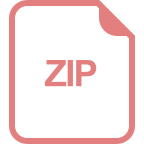
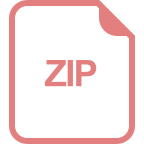
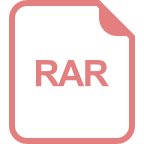
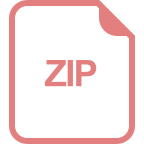
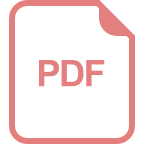
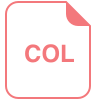
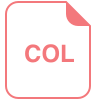
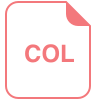







