对于两个输入文件,即文件A和文件B,请编写MapReduce程序,对两个文件进行合并,并剔除其中重复的内容,得到一个新的输出文件C
时间: 2024-04-11 10:33:27 浏览: 10
这是一个典型的MapReduce去重问题。可以采用以下步骤:
1. Map阶段:将文件a和文件b中的每一行作为一个键值对,其中键为行内容,值为一个固定的标记(如1)。
2. Reduce阶段:将Map阶段输出的键值对中的键进行合并,并去除重复的键,最终输出到文件c中。
具体实现可以参考以下代码:
Mapper:
```
public class MergeMapper extends Mapper<Object, Text, Text, IntWritable> {
private final static IntWritable one = new IntWritable(1);
private Text word = new Text();
public void map(Object key, Text value, Context context) throws IOException, InterruptedException {
word.set(value.toString());
context.write(word, one);
}
}
```
Reducer:
```
public class MergeReducer extends Reducer<Text, IntWritable, Text, NullWritable> {
private static final NullWritable nullWritable = NullWritable.get();
public void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException {
context.write(key, nullWritable);
}
}
```
Driver:
```
public class MergeDriver {
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "Merge");
job.setJarByClass(MergeDriver.class);
job.setMapperClass(MergeMapper.class);
job.setReducerClass(MergeReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(IntWritable.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileInputFormat.addInputPath(job, new Path(args[1]));
FileOutputFormat.setOutputPath(job, new Path(args[2]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
以上代码实现了对文件a和文件b的去重合并,并将结果输出到文件c中。
相关推荐
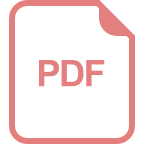




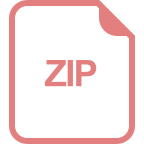
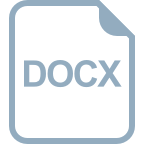
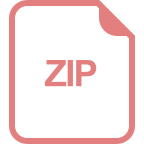
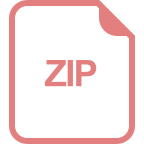
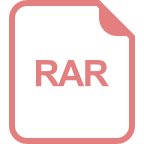
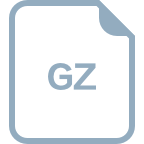
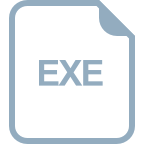