f.write(image) TypeError: a bytes-like object is required, not 'Image'
时间: 2024-09-28 09:09:03 浏览: 33
当你遇到 `TypeError: a bytes-like object is required, not 'Image'` 错误时,这意味着你在尝试将一个 PIL (Pillow) 图像对象 `image` 写入一个二进制文件,但 Python 指出需要的是字节流(bytes-like object)。这是因为文件操作通常需要读取或写入字节而非图像对象。
在处理这种问题时,你需要先将 PIL 图片转换成字节流(通常是 PNG 或 JPEG 格式的二进制数据),然后才能写入文件。以下是一个如何转换并写入文件的例子:
```python
from PIL import Image
import io
# 假设 image 是 PIL 图像对象
image = Image.open('your_image_file.png') # 替换为你的图像路径
# 将图像转换为字节
buffer = io.BytesIO()
image.save(buffer, format='PNG')
image_data = buffer.getvalue() # 现在 you have the byte data
# 然后可以写入文件
with open('screen_shot.png', 'wb') as file:
file.write(image_data)
```
这里首先创建了一个 `BytesIO` 对象,然后使用 `save()` 函数将图像对象保存到这个内存缓冲区,得到的就是字节数据。之后就可以安全地写入文件了。
相关问题
f.write('-----END PGP MESSAGE-----') TypeError: a bytes-like object is required, not 'str'
这个错误通常是因为在写文件时,使用了字符串(str)而不是字节(bytes)类型。为了解决这个问题,你可以将字符串转换为字节串,或者使用以二进制模式打开文件。
以下是将字符串转换为字节串的示例代码:
```
with open('file.txt', 'wb') as f:
f.write(b'Hello, world!')
```
在这个示例中,我们使用了以二进制模式打开文件,使用字节串来写入文件。使用前缀 `b` 可以将字符串转换为字节串。如果你要写入的数据是变量,你可以使用字符串的 `encode()` 方法将其转换为字节串,例如:
```
# 定义字符串
message = 'Hello, world!'
# 将字符串转换为字节串
message_bytes = message.encode('utf-8')
# 以二进制模式写入文件
with open('file.txt', 'wb') as f:
f.write(message_bytes)
```
在这个示例中,我们首先定义了一个字符串变量 `message`,然后使用字符串的 `encode()` 方法将其转换为字节串。最后,我们使用以二进制模式打开文件,并使用字节串写入文件。
TypeError: a bytes-like object is required, not 'JpegImageFile'
这个错误通常是因为尝试对一个 PIL 的 JpegImageFile 对象执行某些操作,但是操作需要的是一个 bytes-like 对象。你需要使用 JpegImageFile 对象的 `tobytes()` 方法将其转换为 bytes-like 对象,然后再进行操作。例如:
```
from PIL import Image
# 打开图片
img = Image.open('example.jpg')
# 将 JpegImageFile 转换为 bytes-like 对象
img_bytes = img.tobytes()
# 现在可以对 img_bytes 进行操作了
```
需要注意的是,`tobytes()` 方法返回的是原始的图像数据,因此在某些情况下可能需要对其进行解码或解压缩。
阅读全文
相关推荐
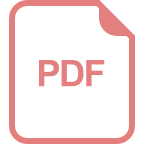
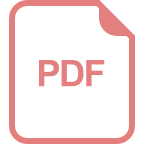











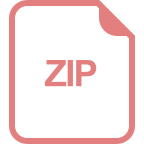
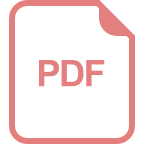
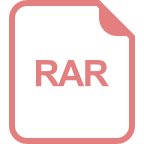