JavaAES 加密解密使用
时间: 2024-06-19 21:03:26 浏览: 113
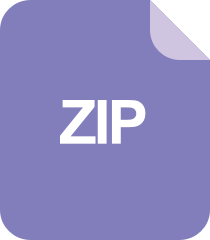
Java AES加密解密
JavaAES加密解密是一种常用的对称加密算法,它可以将明文加密成密文,并且可以使用相同的密钥进行解密。在Java中,常用的JavaAES加密解密实现方式有两种:一种是基于Java自带的Cipher类实现的,另一种是基于第三方库实现的。
如果使用Java自带的Cipher类,可以按照以下步骤实现JavaAES加密解密:
1. 生成密钥,可以使用KeyGenerator类生成密钥。
2. 创建Cipher对象,设置加密模式和填充方式。
3. 初始化Cipher对象,设置加密或解密模式,以及密钥。
4. 调用Cipher的doFinal方法进行加密或解密操作。
以下是JavaAES加密解密的代码示例:
```java
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
import java.nio.charset.StandardCharsets;
import java.security.NoSuchAlgorithmException;
public class JavaAESTest {
public static void main(String[] args) throws Exception {
String plaintext = "Hello, World!"; //明文
String password = "123456"; //密钥
//生成密钥
SecretKey secretKey = getSecretKey(password);
//加密
byte[] ciphertext = encrypt(plaintext.getBytes(StandardCharsets.UTF_8), secretKey);
System.out.println("加密后:" + new String(ciphertext, StandardCharsets.UTF_8));
//解密
byte[] decrypted = decrypt(ciphertext, secretKey);
System.out.println("解密后:" + new String(decrypted, StandardCharsets.UTF_8));
}
private static SecretKey getSecretKey(String password) throws NoSuchAlgorithmException {
//生成AES算法的KeyGenerator对象
KeyGenerator keyGenerator = KeyGenerator.getInstance("AES");
keyGenerator.init(128); //指定密钥长度为128位
//生成一个128位的随机安全密钥
return keyGenerator.generateKey();
}
private static byte[] encrypt(byte[] plaintext, SecretKey secretKey) throws Exception {
//创建Cipher对象,设置加密模式和填充方式
Cipher cipher = Cipher.getInstance("AES/ECB/PKCS5Padding");
cipher.init(Cipher.ENCRYPT_MODE, secretKey); //初始化Cipher对象
//加密并返回结果
return cipher.doFinal(plaintext);
}
private static byte[] decrypt(byte[] ciphertext, SecretKey secretKey) throws Exception {
//创建Cipher对象,设置解密模式和填充方式
Cipher cipher = Cipher.getInstance("AES/ECB/PKCS5Padding");
cipher.init(Cipher.DECRYPT_MODE, secretKey); //初始化Cipher对象
//解密并返回结果
return cipher.doFinal(ciphertext);
}
}
```
阅读全文
相关推荐
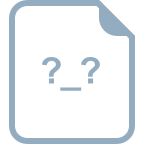


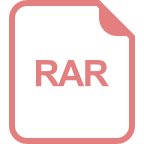
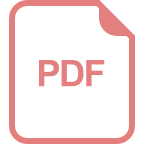
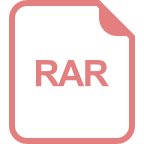
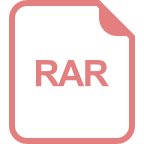
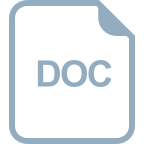
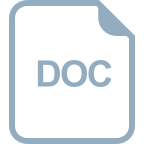
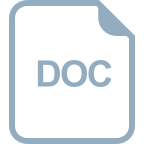
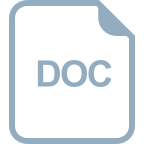
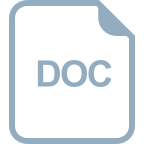

