python代码解决实例
时间: 2024-10-20 07:19:43 浏览: 33
要使用Python代码解决《基于禁忌搜索算法的物流系统车辆路径优化》中的实例,你可以参考以下步骤和代码示例。这个例子将展示如何实现禁忌搜索算法(Tabu Search, TS)来解决带软时间窗的车辆路径问题(VRP with Soft Time Windows, VRPSTW)。
### 1. 导入必要的库
```python
import numpy as np
import random
import matplotlib.pyplot as plt
from itertools import permutations
```
### 2. 定义问题参数
```python
# 分拨中心和客户点的数据
num_customers = 30
depot = {'id': 0, 'x': 35, 'y': 35, 'start_time': 8 * 60, 'end_time': 11.5 * 60, 'service_time': 0}
customers = [
{'id': i, 'x': random.randint(0, 100), 'y': random.randint(0, 100),
'demand': random.randint(100, 500), 'start_time': random.randint(0, 180),
'end_time': random.randint(100, 240), 'service_time': 10} for i in range(1, num_customers + 1)
]
# 车辆参数
num_vehicles = 20
vehicle_capacity = 2000
vehicle_speed = 60 # km/h
vehicle_start_cost = 80 # 元/辆
vehicle_overtime_cost = 50 # 元/小时
early_penalty_cost = 30 # 元/小时
late_penalty_cost = 120 # 元/小时
service_time = 10 # 分钟
max_working_time = 3.5 * 60 # 分钟
```
### 3. 计算距离矩阵
```python
def calculate_distance_matrix(customers):
n = len(customers) + 1 # 包括分拨中心
distance_matrix = np.zeros((n, n))
for i in range(n):
if i == 0:
x1, y1 = depot['x'], depot['y']
else:
x1, y1 = customers[i-1]['x'], customers[i-1]['y']
for j in range(n):
if j == 0:
x2, y2 = depot['x'], depot['y']
else:
x2, y2 = customers[j-1]['x'], customers[j-1]['y']
distance_matrix[i][j] = np.sqrt((x1 - x2)**2 + (y1 - y2)**2)
return distance_matrix
distance_matrix = calculate_distance_matrix(customers)
```
### 4. 初始化禁忌搜索算法
```python
class TabuSearch:
def __init__(self, distance_matrix, customers, num_vehicles, vehicle_capacity, vehicle_start_cost, vehicle_overtime_cost, early_penalty_cost, late_penalty_cost, max_working_time, max_iterations=2000, tabu_tenure=20):
self.distance_matrix = distance_matrix
self.customers = customers
self.num_vehicles = num_vehicles
self.vehicle_capacity = vehicle_capacity
self.vehicle_start_cost = vehicle_start_cost
self.vehicle_overtime_cost = vehicle_overtime_cost
self.early_penalty_cost = early_penalty_cost
self.late_penalty_cost = late_penalty_cost
self.max_working_time = max_working_time
self.max_iterations = max_iterations
self.tabu_tenure = tabu_tenure
self.tabu_list = []
self.best_solution = None
self.best_cost = float('inf')
self.current_solution = self.initialize_solution()
self.current_cost = self.calculate_total_cost(self.current_solution)
def initialize_solution(self):
# 随机初始化解
initial_solution = [list(range(1, len(self.customers) + 1))]
random.shuffle(initial_solution[0])
return initial_solution
def calculate_total_cost(self, solution):
total_cost = 0
for route in solution:
route_cost = 0
current_time = 0
current_load = 0
prev_customer_id = 0 # 分拨中心
for customer_id in route:
customer = self.customers[customer_id - 1]
travel_time = self.distance_matrix[prev_customer_id][customer_id] / vehicle_speed * 60
arrival_time = current_time + travel_time
if arrival_time < customer['start_time']:
waiting_time = customer['start_time'] - arrival_time
route_cost += waiting_time * self.early_penalty_cost
current_time = customer['start_time']
elif arrival_time > customer['end_time']:
delay_time = arrival_time - customer['end_time']
route_cost += delay_time * self.late_penalty_cost
current_time = arrival_time
else:
current_time = arrival_time
current_time += customer['service_time']
current_load += customer['demand']
if current_load > self.vehicle_capacity:
raise ValueError("Exceeded vehicle capacity")
if current_time > self.max_working_time:
route_cost += (current_time - self.max_working_time) * self.vehicle_overtime_cost
route_cost += self.distance_matrix[prev_customer_id][customer_id] * 5 # 单位运输成本
prev_customer_id = customer_id
route_cost += self.distance_matrix[prev_customer_id][0] * 5 # 返回分拨中心的成本
route_cost += self.vehicle_start_cost
total_cost += route_cost
return total_cost
def generate_neighbors(self, solution):
neighbors = []
for i in range(len(solution)):
for j in range(i + 1, len(solution)):
neighbor = solution.copy()
neighbor[i], neighbor[j] = neighbor[j], neighbor[i]
if neighbor not in self.tabu_list:
neighbors.append(neighbor)
return neighbors
def update_tabu_list(self, solution):
if len(self.tabu_list) >= self.tabu_tenure:
self.tabu_list.pop(0)
self.tabu_list.append(solution)
def run(self):
for iteration in range(self.max_iterations):
neighbors = self.generate_neighbors(self.current_solution)
best_neighbor = min(neighbors, key=self.calculate_total_cost)
best_neighbor_cost = self.calculate_total_cost(best_neighbor)
if best_neighbor_cost < self.best_cost:
self.best_solution = best_neighbor
self.best_cost = best_neighbor_cost
self.update_tabu_list(self.current_solution)
self.current_solution = best_neighbor
self.current_cost = best_neighbor_cost
return self.best_solution, self.best_cost
```
### 5. 运行禁忌搜索算法
```python
ts = TabuSearch(distance_matrix, customers, num_vehicles, vehicle_capacity, vehicle_start_cost, vehicle_overtime_cost, early_penalty_cost, late_penalty_cost, max_working_time)
best_solution, best_cost = ts.run()
print(f"Best Solution: {best_solution}")
print(f"Best Cost: {best_cost}")
```
### 6. 可视化结果
```python
def plot_solution(solution, customers, depot):
plt.figure(figsize=(10, 10))
plt.scatter(depot['x'], depot['y'], color='red', label='Depot')
for customer in customers:
plt.scatter(customer['x'], customer['y'], color='blue')
plt.text(customer['x'], customer['y'], f"{customer['id']}")
for route in solution:
x_coords = [depot['x']] + [customers[customer_id - 1]['x'] for customer_id in route] + [depot['x']]
y_coords = [depot['y']] + [customers[customer_id - 1]['y'] for customer_id in route] + [depot['y']]
plt.plot(x_coords, y_coords, color='green')
plt.xlabel('X Coordinate')
plt.ylabel('Y Coordinate')
plt.title('Optimal Delivery Routes')
plt.legend()
plt.show()
plot_solution(best_solution, customers, depot)
```
以上代码展示了如何使用Python实现禁忌搜索算法来解决带软时间窗的车辆路径问题。你可以根据具体需求调整参数和逻辑,以获得更好的解决方案。
阅读全文
相关推荐
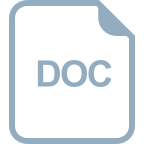
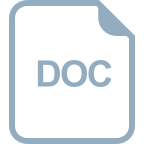
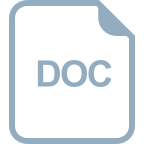
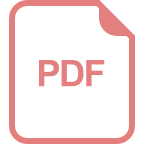
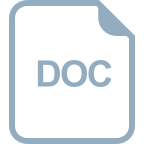
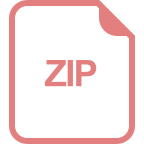
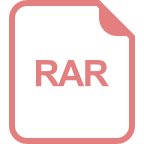
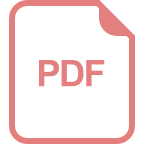
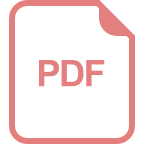
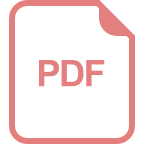
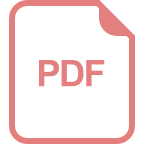
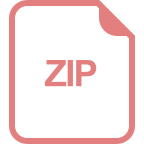
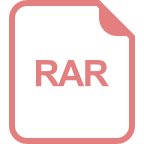
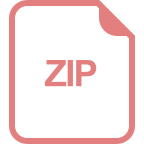
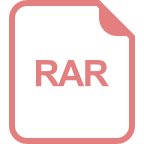
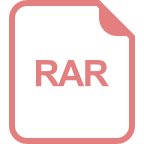
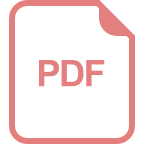
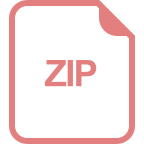
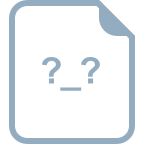