在wxWidgets中怎么让鼠标点击了wxPanel上的区域后在该点生成一个wxBitmapButton对象
时间: 2024-10-25 12:11:13 浏览: 26
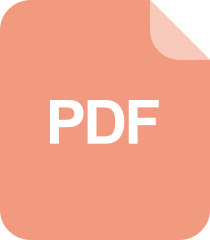
使用wxGLCanvas绘制三维图形-wt901c使用说明书v1.0
在wxWidgets中,要实现在wxPanel上的点击位置动态生成wxBitmapButton对象,你可以采用以下方法:
1. **重写MouseEventType事件**:
首先,重写`wxEVT_MOTION`和`wxEVT_LEFT_UP`这两个鼠标事件,以便捕捉点击和移动事件:
```cpp
class DynamicBitmapButton : public wxPanel
{
DECLARE_EVENT_TABLE()
private:
std::vector<wxBitmapButton*> buttons;
// 其他成员变量...
public:
DynamicBitmapButton(wxWindow* parent, wxWindowID id, const wxPoint& pos = wxDefaultPosition, const wxSize& size = wxDefaultSize, long style = 0);
virtual ~DynamicBitmapButton();
protected:
void OnMotion(wxMouseEvent& event) override;
void OnLeftUp(wxMouseEvent& event) override;
};
```
2. **构造函数**:
在构造函数中初始化一些基本设置:
```cpp
DynamicBitmapButton::DynamicBitmapButton(wxWindow* parent, wxWindowID id, const wxPoint& pos, const wxSize& size, long style)
: wxPanel(parent, id, pos, size, style)
{
// 初始化其他配置...
}
DynamicBitmapButton::~DynamicBitmapButton()
{
// 清理资源...
}
```
3. **事件处理函数**:
- `OnMotion`函数用于跟踪鼠标的位置,如果满足某个条件(如点击阈值),则创建一个新的按钮:
```cpp
void DynamicBitmapButton::OnMotion(wxMouseEvent& event)
{
if (event.LeftIsDown()) {
// 获取鼠标位置
int x = event.GetX();
int y = event.GetY();
// 检查是否在允许区域内或距离已有按钮足够近
if (/* 检查条件 */) {
// 创建新的wxBitmapButton
wxBitmap newBitmap("path_to_new_bitmap.png"); // 替换为你的位图路径
buttons.push_back(new wxBitmapButton(this, -1, newBitmap, wxPoint(x, y), newBitmap.GetSize(), 0));
}
}
}
```
- `OnLeftUp`函数负责在释放鼠标左键时清除旧的按钮,同时添加额外的行为(如移除已点击的按钮):
```cpp
void DynamicBitmapButton::OnLeftUp(wxMouseEvent& event)
{
if (event.LeftIsDown())
{
for (auto& button : buttons)
{
if (button->GetPosition() == event.GetPosition()) { /* 处理点击事件 */ break; } // 可能需要移除按钮或执行其他操作
}
}
}
}
4. **清理资源**:
在类析构函数中,确保正确删除所有动态创建的wxBitmapButton实例,以避免内存泄漏。
注意:在实际应用中,你可能需要调整判断条件和事件处理逻辑,使其更适应你的需求。
阅读全文
相关推荐
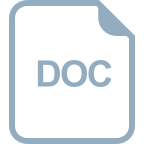
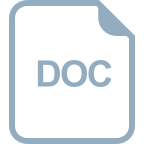

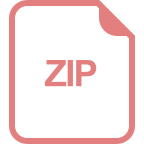
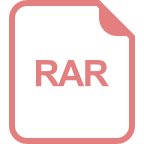
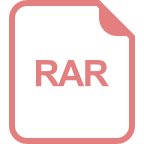
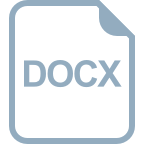
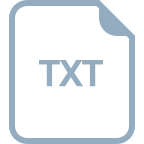
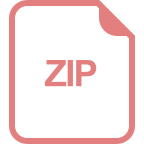
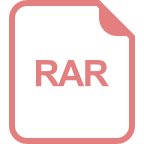
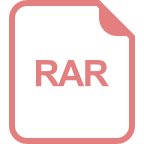
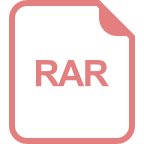



