在pyrhon中给定一个句子,只包含字母和空格,将句子中的单词位置反转并且首字母大写
时间: 2024-10-13 12:13:30 浏览: 32
在Python中,你可以通过以下步骤来实现这个功能:
1. 首先,你需要将输入的句子按照空格分割成单词列表。
2. 然后,对这个列表进行反转,即将单词的位置颠倒过来。
3. 对于每个单词,使用字符串的capitalize()方法使其首字母大写。
4. 最后,使用join()函数把单词连接回原始句子。
下面是一个示例代码片段来演示如何操作:
```python
def reverse_words(sentence):
# 分割句子成单词列表
words = sentence.split(' ')
# 反转单词列表
reversed_words = words[::-1]
# 首字母大写并重新组合句子
reversed_sentence = ' '.join(word.capitalize() for word in reversed_words)
return reversed_sentence
# 示例
sentence = "hello world this is python"
reversed_and_capitalized = reverse_words(sentence)
print(reversed_and_capitalized) # 输出: "Python Is This World Hello"
```
相关问题
C语言给定一个字符串把其中各个单词的顺序颠倒,注意保持句首字母大写,标点符号不动。
可以使用以下步骤实现:
1. 定义一个函数,接受一个字符串参数,返回一个字符串结果。
2. 定义一个数组,存储每个单词的起始位置和长度。
3. 遍历字符串,找到每个单词的起始位置和长度,并将其存储到数组中。
4. 遍历数组,将每个单词反转。
5. 遍历数组,将每个单词的首字母大写。
6. 遍历数组,将每个单词连接起来,中间加上空格。
下面是实现该函数的代码:
```c
#include <ctype.h>
#include <string.h>
char* reverseWords(char* s) {
int len = strlen(s);
int words[100][2];
int count = 0;
int i = 0;
while (i < len) {
while (i < len && isspace(s[i])) i++;
if (i == len) break;
words[count][0] = i;
while (i < len && !isspace(s[i])) i++;
words[count][1] = i - words[count][0];
count++;
}
for (int i = 0; i < count; i++) {
int start = words[i][0];
int end = words[i][0] + words[i][1] - 1;
while (start < end) {
char tmp = s[start];
s[start] = s[end];
s[end] = tmp;
start++;
end--;
}
}
for (int i = 0; i < count; i++) {
int idx = words[i][0];
s[idx] = toupper(s[idx]);
}
char* result = malloc(len + 1);
memset(result, 0, len + 1);
for (int i = 0; i < count; i++) {
int start = words[i][0];
strncat(result, s + start, words[i][1]);
if (i < count - 1) strcat(result, " ");
}
return result;
}
```
注意,该函数返回的字符串需要手动释放。可以这样调用:
```c
char* s = "Hello, world!";
char* result = reverseWords(s);
printf("%s\n", result);
free(result);
```
C语言,给定一个字符串把其中各个单词的顺序颠倒,注意保持句首字母大写,标点符号不动。
可以使用以下步骤实现:
1. 定义一个函数,接受一个字符串参数,返回一个字符串结果。
2. 定义两个指针,分别指向字符串的开头和结尾。
3. 从结尾向开头遍历字符串,找到每个单词的结尾位置。
4. 再从每个单词的结尾位置向开头遍历,将每个单词反转。
5. 遍历字符串,将每个单词的首字母大写。
6. 遍历字符串,将每个单词连接起来,中间加上空格。
下面是实现该函数的代码:
```c
#include <ctype.h>
#include <string.h>
char* reverseWords(char* s) {
int len = strlen(s);
char* start = s;
char* end = s + len - 1;
while (isspace(*end)) end--;
char* word_end = end;
while (word_end >= start) {
if (isspace(*word_end)) {
int word_len = end - word_end;
char* word_start = word_end + 1;
for (char* p = word_start; p < end; p++) {
char tmp = *p;
*p = *word_end;
*word_end = tmp;
word_end--;
}
end = word_end;
word_end -= word_len + 1;
} else {
word_end--;
}
}
for (char* p = s; p <= end; p++) {
if (p == s || isspace(*(p - 1))) {
*p = toupper(*p);
}
}
char* result = malloc(len + 1);
memset(result, 0, len + 1);
char* p = s;
while (p <= end) {
if (!isspace(*p)) {
strncat(result, p, 1);
} else if (p > s && !isspace(*(p - 1))) {
strcat(result, " ");
}
p++;
}
return result;
}
```
注意,该函数返回的字符串需要手动释放。可以这样调用:
```c
char* s = "Hello, world!";
char* result = reverseWords(s);
printf("%s\n", result);
free(result);
```
阅读全文
相关推荐
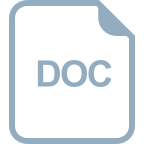
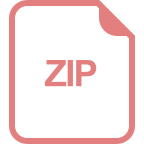
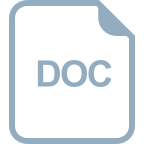
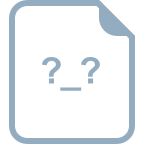
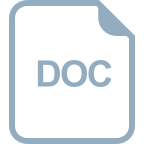
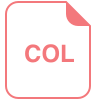
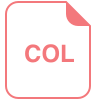
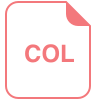








