python 命名实体识别算法
时间: 2023-11-18 13:56:24 浏览: 42
Python命名实体识别算法是一种自然语言处理技术,用于从文本中识别出具有特定意义的实体,例如人名、地名、组织机构名、日期、时间等。该算法可以通过使用正则表达式、机器学习和深度学习等技术来实现。在Python中,常用的命名实体识别算法包括NLTK、spaCy、Stanford NER等。这些算法可以帮助我们更好地理解文本中的实体,并为后续的自然语言处理任务提供更好的基础。
相关问题
python 命名实体识别
Python中有许多用于命名实体识别(Named Entity Recognition,NER)的库和工具包。以下是几个常用的库:
1. spaCy:spaCy是一个流行的自然语言处理(NLP)库,提供了强大的NER功能。它支持多种预训练的模型,并且易于使用和扩展。
2. NLTK:Natural Language Toolkit(NLTK)是Python中常用的NLP库之一。它提供了一些NER算法和模型,可以用于识别命名实体。
3. Stanford NER:Stanford NER是斯坦福大学开发的命名实体识别工具。Python中可以使用NLTK库来集成Stanford NER。
4. Flair:Flair是一个用于NLP任务的强大框架,包括NER。它提供了预训练的模型,可以直接用于NER任务。
这些库提供了各种算法和模型,你可以根据自己的需求选择适合的库来进行命名实体识别。
python 实体命名识别
在 Python 中,实体命名识别(Named Entity Recognition,NER)可以使用一些开源工具和库来实现。以下是一些常用的工具和库:
1. spaCy:spaCy 是一个流行的自然语言处理库,其中包含了 NER 功能。它可以轻松地识别人名、组织名、地名等实体,并且支持多语言。
2. NLTK:NLTK 是另一个常用的自然语言处理库,它也提供了 NER 功能。NLTK 的 NER 模块使用基于规则的方法进行实体识别。
3. Stanford NER:Stanford NER 是一个 Java 实现的实体命名识别工具,但是它也提供了一个 Python 接口。Stanford NER 使用了最新的机器学习算法,并且支持多种实体类型。
4. Flair:Flair 是一个用于自然语言处理的 Python 库,它提供了各种 NLP 功能,包括实体命名识别。Flair 的 NER 模块基于深度学习方法,可以实现高精度的实体识别。
这些工具和库都可以通过 pip 安装,使用起来也比较简单。你可以根据自己的需求选择其中一个进行实体命名识别。
相关推荐
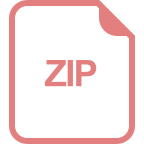
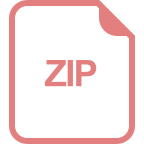
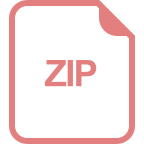
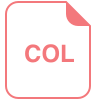
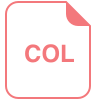
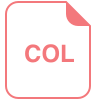
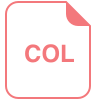
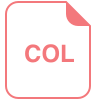







