C语音20以内加减乘除自动输出,并计算正确率
时间: 2024-12-15 10:13:38 浏览: 4
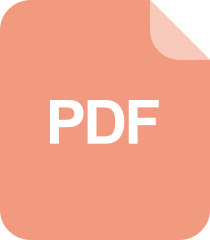
C语言实现矩阵的加减乘除求逆运算.pdf
在C语言中实现一个简单的加减乘除自动计算器,并统计正确率,你需要编写一个控制循环的程序,接收用户输入的数字和操作符,然后进行相应的计算。这里是一个基础的例子:
```c
#include <stdio.h>
// 函数声明
int add(int a, int b);
int subtract(int a, int b);
int multiply(int a, int b);
int divide(int a, int b);
int main() {
int correct_count = 0;
int total_attempts = 0;
while (1) {
printf("\nEnter an operation (1 for addition, 2 for subtraction, 3 for multiplication, or 4 for division): ");
int choice;
scanf("%d", &choice);
if (choice < 1 || choice > 4) {
printf("Invalid choice. Please try again.\n");
continue;
}
printf("Enter the first number: ");
int num1;
scanf("%d", &num1);
printf("Enter the second number: ");
int num2;
scanf("%d", &num2);
switch (choice) {
case 1:
int result = add(num1, num2);
break;
case 2:
result = subtract(num1, num2);
break;
case 3:
result = multiply(num1, num2);
break;
case 4:
if (num2 == 0) {
printf("Error: Division by zero is not allowed.\n");
continue;
}
result = divide(num1, num2);
break;
}
if (choice == 1 && result == num1 + num2) {
correct_count++;
} else if (choice == 2 && result == num1 - num2) {
correct_count++;
} else if (choice == 3 && result == num1 * num2) {
correct_count++;
} else if (choice == 4 && !((num2 != 0) && (result == num1 / num2))) {
correct_count++; // 注意这里的判断,因为除法结果可能有小数部分,此处仅作为演示
}
total_attempts++;
printf("Result: %d\n", result);
printf("Correct rate: %.2f%%\n", (correct_count * 100.0) / total_attempts);
printf("Do you want to continue? (y/n): ");
char cont;
scanf(" %c", &cont);
if (cont != 'y' && cont != 'Y') {
break;
}
}
return 0;
}
// 定义实际的加、减、乘、除函数
int add(int a, int b) { return a + b; }
int subtract(int a, int b) { return a - b; }
int multiply(int a, int b) { return a * b; }
int divide(int a, int b) { return a / b; }
```
这个示例中,我们首先询问用户的操作选择和数字,然后根据选择进行计算并比较结果。如果计算结果正确,就增加正确计数。最后,我们会显示当前的正确率,并询问用户是否继续。注意,此示例仅用于演示目的,实际应用中可能需要考虑更多的边界条件和浮点数处理。
阅读全文
相关推荐
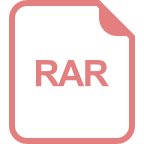















