cad判断两条线相交,返回交点c#
时间: 2024-09-11 21:07:11 浏览: 70
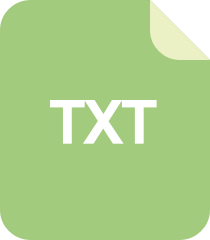
判断两线段是否相交,相交求交点

在CAD软件中,判断两条线段是否相交并返回交点通常涉及几何计算。在C#中,这通常需要使用CAD软件提供的API或者SDK来实现。以AutoCAD为例,你可以使用AutoCAD的.NET API来进行这样的判断和计算。以下是一个简化的代码示例,展示了如何使用AutoCAD的.NET API来判断两条直线是否相交并获取交点:
```csharp
using Autodesk.AutoCAD.Runtime;
using Autodesk.AutoCAD.ApplicationServices;
using Autodesk.AutoCAD.DatabaseServices;
using Autodesk.AutoCAD.Geometry;
// 这是一个AutoCAD命令的示例
public class IntersectLineCommands
{
// 该方法用于判断两条直线是否相交
[CommandMethod("IntersectLines")]
public void IntersectTwoLines()
{
Document acDoc = Application.DocumentManager.MdiActiveDocument;
Database acCurDb = acDoc.Database;
// 开始一个事务
using (Transaction acTrans = acCurDb.TransactionManager.StartTransaction())
{
// 打开块表记录用于读写
BlockTable acBlkTbl;
acBlkTbl = acTrans.GetObject(acCurDb.BlockTableId, OpenMode.ForRead) as BlockTable;
// 打开块表记录模型空间用于写入
BlockTableRecord acBlkTblRec;
acBlkTblRec = acTrans.GetObject(acBlkTbl[BlockTableRecord.ModelSpace], OpenMode.ForWrite) as BlockTableRecord;
// 创建两条直线作为示例
Line acLine1 = new Line(new Point3d(0, 0, 0), new Point3d(10, 10, 0));
Line acLine2 = new Line(new Point3d(0, 10, 0), new Point3d(10, 0, 0));
// 将新对象添加到块表记录和事务
acBlkTblRec.AppendEntity(acLine1);
acTrans.AddNewlyCreatedDBObject(acLine1, true);
acBlkTblRec.AppendEntity(acLine2);
acTrans.AddNewlyCreatedDBObject(acLine2, true);
// 判断两条线段是否相交
Point3dCollection intersectionPoints = new Point3dCollection();
acLine1.IntersectWith(acLine2, Intersect.OnBothOperands, intersectionPoints, IntPtr.Zero, IntPtr.Zero);
if (intersectionPoints.Count > 0)
{
// 如果有交点,则输出交点坐标
acDoc.Editor.WriteMessage("交点坐标: " + intersectionPoints[0].ToString() + "\n");
}
else
{
// 如果没有交点,则输出提示信息
acDoc.Editor.WriteMessage("两条线段不相交。\n");
}
// 提交事务
acTrans.Commit();
}
}
}
```
需要注意的是,上述代码仅作为示例,并未考虑所有可能的异常处理和用户交互逻辑。在实际使用中,你需要根据实际CAD软件的具体API文档来编写代码,并确保处理好所有可能的异常情况。
阅读全文
相关推荐
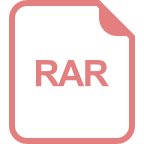
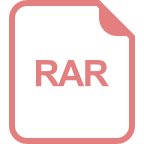
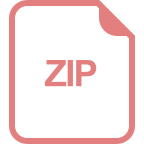
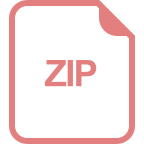
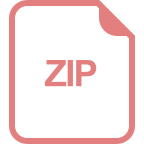
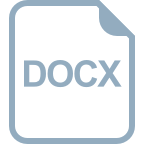
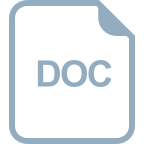
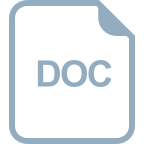
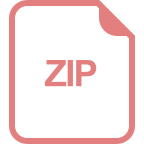
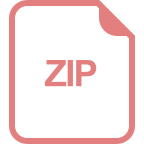
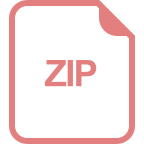
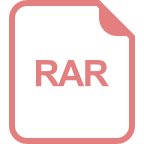
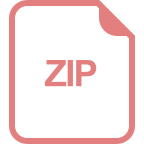
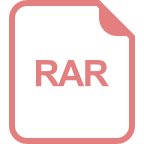