springboot+vue购物车
时间: 2023-12-04 17:04:11 浏览: 142
基于Spring Boot和Vue的购物车实现可以分为以下几个步骤:
1. 创建商品列表页面,展示所有商品信息,包括商品名称、价格、图片等,并在每个商品后面添加“加入购物车”按钮。
2. 点击“加入购物车”按钮后,将该商品信息添加到购物车中。可以使用VueX来实现购物车的状态管理。
3. 创建购物车页面,展示当前购物车中的所有商品信息,包括商品名称、价格、数量、小计等,并在每个商品后面添加“删除”按钮和“增加/减少数量”按钮。
4. 点击“删除”按钮后,将该商品从购物车中删除。
5. 点击“增加/减少数量”按钮后,可以增加或减少该商品的数量,并更新小计金额。
6. 在购物车页面中添加“结算”按钮,点击后跳转到订单确认页面。
下面是一个简单的示例代码,仅供参考:
1. 商品列表页面
```vue
<template>
<div>
<div v-for="item in productList" :key="item.id">
<img :src="item.image" alt="">
<p>{{ item.name }}</p>
<p>{{ item.price }}</p>
<button @click="addToCart(item)">加入购物车</button>
</div>
</div>
</template>
<script>
export default {
data() {
return {
productList: [
{
id: 1,
name: '商品1',
price: 100,
image: 'https://xxx.com/1.jpg'
},
{
id: 2,
name: '商品2',
price: 200,
image: 'https://xxx.com/2.jpg'
},
// 其他商品信息
]
}
},
methods: {
addToCart(item) {
this.$store.commit('addToCart', item)
}
}
}
</script>
```
2. 购物车页面
```vue
<template>
<div>
<div v-for="item in cartList" :key="item.id">
<img :src="item.image" alt="">
<p>{{ item.name }}</p>
<p>{{ item.price }}</p>
<button @click="decreaseQuantity(item)">-</button>
<span>{{ item.quantity }}</span>
<button @click="increaseQuantity(item)">+</button>
<p>{{ item.subtotal }}</p>
<button @click="removeFromCart(item)">删除</button>
</div>
<p>总计:{{ total }}</p>
<button @click="checkout">结算</button>
</div>
</template>
<script>
export default {
computed: {
cartList() {
return this.$store.state.cartList
},
total() {
let sum = 0
this.cartList.forEach(item => {
sum += item.subtotal
})
return sum
}
},
methods: {
removeFromCart(item) {
this.$store.commit('removeFromCart', item)
},
increaseQuantity(item) {
this.$store.commit('increaseQuantity', item)
},
decreaseQuantity(item) {
this.$store.commit('decreaseQuantity', item)
},
checkout() {
// 跳转到订单确认页面
}
}
}
</script>
```
3. VueX状态管理
```javascript
const store = new Vuex.Store({
state: {
cartList: []
},
mutations: {
addToCart(state, item) {
const index = state.cartList.findIndex(i => i.id === item.id)
if (index === -1) {
state.cartList.push({
...item,
quantity: 1,
subtotal: item.price
})
} else {
state.cartList[index].quantity++
state.cartList[index].subtotal += item.price
}
},
removeFromCart(state, item) {
const index = state.cartList.findIndex(i => i.id === item.id)
state.cartList.splice(index, 1)
},
increaseQuantity(state, item) {
const index = state.cartList.findIndex(i => i.id === item.id)
state.cartList[index].quantity++
state.cartList[index].subtotal += item.price
},
decreaseQuantity(state, item) {
const index = state.cartList.findIndex(i => i.id === item.id)
if (state.cartList[index].quantity > 1) {
state.cartList[index].quantity--
state.cartList[index].subtotal -= item.price
}
}
}
})
```
阅读全文
相关推荐
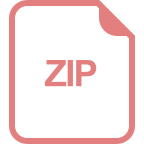

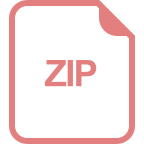
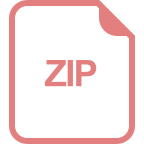
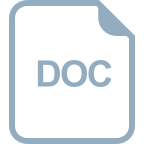
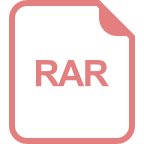










