android studio com.google.android.material.tabs.TabLayout如何添加选中图片
时间: 2024-09-14 09:15:03 浏览: 97
在Android Studio中,使用`com.google.android.material.tabs.TabLayout`组件时,如果想要为每个tab添加选中的图片,可以通过创建一个自定义的tab布局来实现。以下是一个简单的示例:
1. 创建一个自定义的tab布局XML文件(例如`custom_tab.xml`),在这个布局文件中,你可以放置ImageView作为选中时的图片,以及其他视图元素。
```xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical"
android:gravity="center"
android:background="?attr/selectableItemBackgroundBorderless">
<ImageView
android:id="@+id/tab_image"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/ic_unselected_tab" />
<TextView
android:id="@+id/tab_text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Tab Name" />
</LinearLayout>
```
2. 在你的Activity或Fragment中设置TabLayout时,使用`TabLayout`的`addTab`方法,并为每个tab设置自定义视图。
```java
TabLayout tabLayout = findViewById(R.id.tabLayout);
TabLayout.Tab tab = tabLayout.newTab();
tab.setCustomView(R.layout.custom_tab);
// 设置选中和未选中时的图片资源
ImageView tabImageView = tab.getCustomView().findViewById(R.id.tab_image);
tabImageView.setImageResource(R.drawable.ic_selected_tab);
tab.setText("Tab Name");
tabLayout.addTab(tab);
```
3. 为了在tab被选中时更换图片,你可以设置`TabLayout`的`addOnTabSelectedListener`监听器。
```java
tabLayout.addOnTabSelectedListener(new TabLayout.OnTabSelectedListener() {
@Override
public void onTabSelected(TabLayout.Tab tab) {
ImageView tabImageView = tab.getCustomView().findViewById(R.id.tab_image);
tabImageView.setImageResource(R.drawable.ic_selected_tab);
}
@Override
public void onTabUnselected(TabLayout.Tab tab) {
ImageView tabImageView = tab.getCustomView().findViewById(R.id.tab_image);
tabImageView.setImageResource(R.drawable.ic_unselected_tab);
}
@Override
public void onTabReselected(TabLayout.Tab tab) {
// 可以在这里处理tab重新被选中的事件
}
});
```
请确保你已经将所需的图片资源添加到你的res/drawable文件夹中,并且根据你的布局和需求适当地调整上述代码。
阅读全文
相关推荐





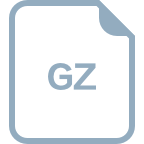












