将浮点数类型转换成字符串,如果有小数则保留小数字符串,小数位为0则保留整数字符串
时间: 2024-11-16 07:03:03 浏览: 8
在JavaScript中,你可以使用`toFixed()`方法将浮点数转换为字符串,并指定保留的小数位数。例如:
```javascript
let num = 42.00;
let strWithDecimal = num.toFixed(2); // 结果是 "42.00"
let strWithoutDecimal = Math.trunc(num).toString(); // 结果是 "42"
if (strWithDecimal !== strWithoutDecimal) {
console.log(strWithDecimal); // 如果有小数,保留小数部分
} else {
console.log(strWithoutDecimal); // 如果小数位为0,只保留整数部分
}
```
`toFixed()`会返回一个字符串,包括指定的小数位,如果没有小数则会在末尾添加零。而`Math.trunc()`函数用于去掉小数部分,得到整数值。
注意,`toFixed()`在某些浏览器下并不总是精确的,对于较大数值的精确控制,可以考虑使用第三方库,如`decimal.js`等。
相关问题
Java 将浮点数类型转换成字符串,如果有小数则保留小数字符串,小数位为0则保留整数字符串
在Java中,将浮点数类型转换为字符串,通常可以使用`String`类的`valueOf()`方法结合`DecimalFormat`或`NumberFormat`来进行格式化。如果你想保留固定的小数位,可以使用`DecimalFormat`的`setMaximumFractionDigits()`方法来设置最多显示几位小数;如果小数位为0,你不需要特别处理,`valueOf()`会自动省略。
例如,下面是一个例子:
```java
import java.text.DecimalFormat;
public class Main {
public static void main(String[] args) {
double num = 3.14;
DecimalFormat df = new DecimalFormat("#.##");
df.setMaximumFractionDigits(2); // 设置保留两位小数
String strNum = df.format(num);
System.out.println(strNum); // 输出 "3.14"
// 如果数字本身没有小数,如 num = 5,则输出 "5",自动去掉小数部分
}
}
```
在这个示例中,无论`num`有多少位小数,`format()`方法都会将其转换为字符串,并保留指定的最大小数位。
C++实现浮点数转字符串并保留一位小数位并四舍五入
在C++中,你可以使用`std::stringstream`和`std::fixed`配合`std::setprecision`来实现浮点数转换成字符串,并保留一位小数并四舍五入。以下是一个简单的示例:
```cpp
#include <iostream>
#include <sstream>
#include <iomanip>
// 函数模板用于将float转换为保留一位小数的字符串
template<typename T>
std::string to_string_with_precision(T num, int precision) {
std::stringstream ss;
ss << std::fixed << std::setprecision(precision) << num;
return ss.str();
}
int main() {
float f = 3.141592653589793;
std::string result = to_string_with_precision(f, 1);
std::cout << "Float number with one decimal place after rounding: " << result << std::endl;
return 0;
}
```
在这个例子中,`to_string_with_precision`函数接受一个float类型的数值和一个整数精度作为参数。`std::fixed`确保了数字是以固定小数点形式显示,`std::setprecision(1)`则设置只保留一位小数。
阅读全文
相关推荐
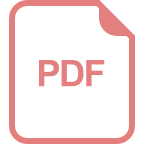
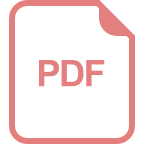
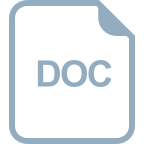
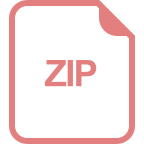
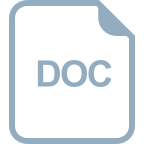










