用状态机的程序设计方法设计一个按键实现停止状态、手动状态、自动状态的切换的代码
时间: 2024-10-22 16:23:37 浏览: 8
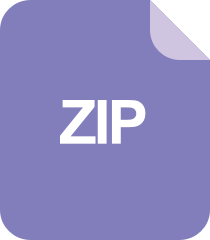
stm32 按键状态机扫描

在程序设计中,可以使用状态机(State Machine)的概念来管理按键控制的不同状态,如停止(Stop)、手动(Manual)和自动(Automatic)。这里我们假设有一个名为`KbController`的状态类,它包含三个内部状态(StopState, ManualState, AutomaticState),并有一个外部接口用于处理按键事件。
首先,创建三个状态类:
```python
class StopState:
def handle_button_press(self):
print("按下按钮,尝试转换到手动状态")
class ManualState:
def handle_button_press(self):
if self.is_allowed_to_change():
self.controller.change_state(AutomaticState)
print("切换到自动状态")
else:
print("手动模式下无法直接转到自动")
class AutomaticState:
def handle_button_press(self):
print("在自动状态下,忽略按键")
class KbController:
_current_state = None
def __init__(self):
self._current_state = StopState()
def change_state(self, new_state):
self._current_state = new_state
self._current_state.handle_button_press()
# 其他辅助方法,比如检查是否允许状态转换等...
```
在这个例子中,初始化`KbController`时设置为停止状态。当按钮被按下时,`handle_button_press`方法会被当前状态调用。如果在手动状态下,只有在满足条件(例如安全检查)时才会转换到自动状态。在自动状态下则不会响应按键。
为了实现上述功能,你可以添加一个外部接口函数来开始按键操作:
```python
def start_kb_controller():
while True:
# 模拟按键输入
button_pressed = input("模拟按键,按回车:").lower()
if button_pressed == "start":
break
kb_controller = KbController()
while True:
kb_controller.handle_button_press()
```
阅读全文
相关推荐
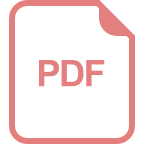
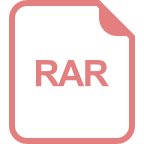
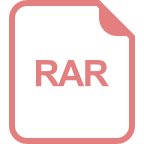
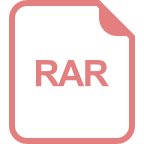
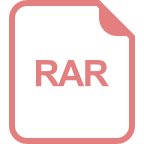
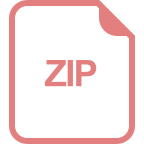
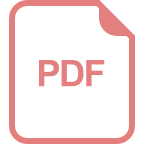
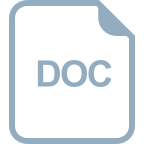
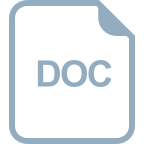
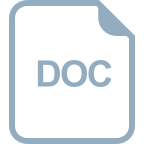
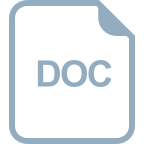
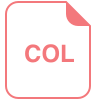
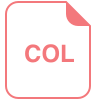



