css自定义组件类名前缀
时间: 2024-03-14 18:41:49 浏览: 242
自定义组件类名前缀可以通过修改CSS样式表或使用CSS预处理器来实现。以下是两种方法:
1. 修改CSS样式表:[^1]
```css
/* 在样式表中添加自定义组件类名前缀 */
.custom-prefix-button {
/* 样式规则 */
}
.custom-prefix-card {
/* 样式规则 */
}
/* 使用自定义组件类名前缀 */
<div class="custom-prefix-button">按钮</div>
<div class="custom-prefix-card">卡片</div>
```
2. 使用CSS预处理器(例如Sass):[^2]
```scss
// 在Sass文件中定义变量
$component-prefix: "custom-prefix-";
// 使用变量来生成自定义组件类名前缀
.#{$component-prefix}button {
/* 样式规则 */
}
.#{$component-prefix}card {
/* 样式规则 */
}
// 编译后的CSS将包含自定义组件类名前缀
<div class="custom-prefix-button">按钮</div>
<div class="custom-prefix-card">卡片</div>
```
相关问题
react项目中如何给所有antd组件加上前缀类名
在React项目中,如果你想要给所有Ant Design (antd) 组件添加前缀类名以保持样式管理的统一,可以按照以下步骤操作:
1. **设置全局主题**:
- 首先,在`antd`的配置文件中,通常是在`src/theme.js`或`.less`文件中,你可以创建一个自定义的主题,并将所有的`className`前缀设置为你需要的名称。例如:
```javascript
import { createTheme } from 'antd';
const prefixCls = 'your-prefix'; // 自定义前缀
const theme = createTheme({
components: {
antd: {
prefixCls,
},
},
});
```
然后,你需要在项目的其他部分引入并应用这个主题。
2. **使用高阶组件(HOC)**:
- 创建一个HOC( Higher-Order Component),它接收一个组件作为参数,修改它的props,如添加前缀到CSS类名。例如:
```jsx
function WithPrefix(Component) {
return function WithPrefixWrapper(props) {
const { className, ...rest } = props;
return <Component prefixCls={prefixCls} className={`${prefixCls}-${className}`} {...rest} />;
};
}
```
3. **应用HOC**:
- 将这个HOC应用到所有你想加前缀的antd组件上,比如按钮、表单等:
```jsx
<WithPrefix<Button type="primary">点击</Button>
```
通过以上方法,所有antd组件都会自动带上你设置的前缀类名。然而,这可能会导致样式冲突,所以建议在设计时尽量避免直接覆盖核心ant design的样式。
vue自定义elementui前缀
### 如何在 Vue 中设置 ElementUI 组件自定义前缀
为了实现在 Vue 项目中给 ElementUI 组件添加自定义前缀,可以采用多种方式来满足不同的需求场景。
#### 方法一:全局配置命名空间
如果希望为所有的 ElementUI 组件统一增加一个特定的类名作为前缀,可以在安装 `Element` 插件的时候通过修改其 CSS 变量或者利用 BEM 命名约定来进行处理。不过更简便的方式是在引入 Element UI 后,在 main.js 或者入口文件里对 `$ELEMENT` 进行扩展:
```javascript
import Vue from 'vue';
import ElementUI from 'element-ui';
import locale from 'element-ui/lib/locale/lang/en'; // 如果需要国际化支持则导入相应语言包
Vue.use(ElementUI, {
size: 'small', // 默认尺寸
zIndex: 3000,
});
// 添加自定义前缀到 $ELEMENT 对象上
Vue.prototype.$ELEMENT = Object.assign({}, locale, {
prefixCls: 'my-prefix-' // 此处设定想要使用的前缀名称
});
```
这会使得所有由 ElementUI 渲染出来的 HTML 元素都会带有指定的前缀类名[^1]。
#### 方法二:局部作用域内调整单个组件样式
对于只需要针对某个具体页面或模块下的个别组件应用特殊样式的场合,则可以直接在模板内部操作。比如要改变 Tree 组件前面的小图标,可以通过如下方式进行定制化:
```html
<template>
<el-tree :data="data" node-key="id">
<!-- 使用 scoped slot 来覆盖原有结构 -->
<span class="custom-icon-slot" slot-scope="{node}">
<i v-if="!node.isLeaf && !expandedKeys.includes(node.key)" @click.stop="toggleExpand(node)">
<svg aria-hidden="true"><use xlink:href="#icon-right"></use></svg> <!-- 自定义关闭状态图标 -->
</i>
<i v-else-if="!node.isLeaf && expandedKeys.includes(node.key)" @click.stop="toggleExpand(node)">
<svg aria-hidden="true"><use xlink:href="#icon-down"></use></svg> <!-- 自定义打开状态图标 -->
</i>
{{ node.label }}
</span>
</el-tree>
</template>
<script>
export default {
data() {
return {
data: [...], // tree 数据源
expandedKeys: [] // 记录当前已展开节点 id 列表
};
},
methods:{
toggleExpand(node){
const index=this.expandedKeys.indexOf(node.key);
if(index>-1)this.expandedKeys.splice(index,1);else this.expandedKeys.push(node.key);
}
}
};
</script>
<style lang="scss" scoped>
.custom-icon-slot i{
cursor:pointer;
}
/* 需要在自己的项目中有对应的 svg sprite 图片资源 */
@font-face {
font-family:'iconfont';
src:url('//at.alicdn.com/t/font_8d73422fwewfewe.ttf') format('truetype');
}
.icon-right,.icon-down{ /* 定义两个图标的样式 */
display:inline-block;width:1em;height:1em;fill:#ccc;font-size:.9rem;
}
.icon-right::before,.icon-down::before{/* 引入阿里云字体库中的 icon*/
content:"";
background:url("//at.alicdn.com/t/font_8d73422fwefwe.png") no-repeat center / contain ;
}
.icon-right{transform:rotate(0deg);}
.icon-down{transform:rotate(-90deg);}
</style>
```
上述代码片段展示了如何创建一个新的插槽来自定义树形控件每项之前显示的内容,并且提供了两种不同状态下切换显示的不同方向箭头效果。需要注意的是这里的 SVG 和 Font Icon 是假设性的例子,实际使用时应替换为自己项目的图形资产路径[^3]。
阅读全文
相关推荐




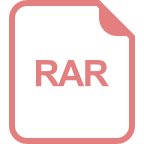











