已知某节目采用计票机制,选手获得的票数越多,排名越靠前。要求编写程序,接收选手的姓名和票数,输出排序后的成绩
时间: 2024-12-01 12:29:07 浏览: 0
为了实现这样一个程序,我们可以使用Python的数据结构,比如列表或字典来存储选手的信息,并利用内置的`sorted()`函数来进行排序。下面是一个简单的例子:
```python
class Contestant:
def __init__(self, name, votes):
self.name = name
self.votes = votes
def sort_contestants(contestants):
# 使用元组作为元素,元组的第一个元素是票数,第二个是名字,这样可以按照票数降序排序
sorted_contestants = sorted(contestants, key=lambda x: (-x.votes, x.name))
return sorted_contestants
# 示例数据
contestants = [
Contestant("选手A", 50),
Contestant("选手B", 75),
Contestant("选手C", 45),
Contestant("选手D", 80)
]
# 排序并输出结果
results = sort_contestants(contestants)
for i, contestant in enumerate(results, start=1):
print(f"{i}. {contestant.name}: {contestant.votes} 票")
# --
相关问题
已知某节目采用机票机制,选手票数越多排名越靠前,编写程序,接收选手的姓名和票数,输出排序后的成绩
在Python中,你可以使用列表和字典结合的方式来模拟这种机票机制并排序。首先,可以创建一个字典存储每个选手及其票数,然后通过排序函数将选手按照票数从高到低排列。以下是一个简单的示例:
```python
def ticket_sort(competitors):
# 创建字典,键是选手名,值是票数
votes = {name: vote for name, vote in competitors.items()}
# 按照票数降序排序
sorted_competitors = dict(sorted(votes.items(), key=lambda item: item[1], reverse=True))
return sorted_competitors
# 示例输入
competitors = {"选手A": 500, "选手B": 400, "选手C": 600}
sorted_scores = ticket_sort(competitors)
for name, score in sorted_scores.items():
print(f"{name}: {score} 票")
#
已知某节目采用计票机制,选手获得的票数越多,排名就越靠前。要求用Python编写程序,接收选手的姓名和票数,输出排序后的成绩。
为了实现这个功能,你可以使用Python的内置数据结构`list`或`dict`来存储选手及其票数,然后通过遍历并比较票数对列表进行排序。这里是一个简单的示例:
```python
class VoteCounter:
def __init__(self):
self.votes = {}
def add_vote(self, name, votes):
if name in self.votes:
self.votes[name] += votes
else:
self.votes[name] = votes
def sort_by_votes(self):
sorted_votes = sorted(self.votes.items(), key=lambda x: x[1], reverse=True)
return sorted_votes
# 使用示例
counter = VoteCounter()
counter.add_vote('张三', 500)
counter.add_vote('李四', 700)
counter.add_vote('王五', 300)
sorted_scores = counter.sort_by_votes()
for i, (name, votes) in enumerate(sorted_scores, start=1):
print(f"第{i}名:{name},得票数:{votes}")
#
阅读全文
相关推荐
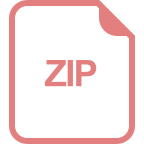
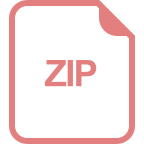
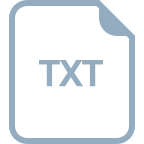



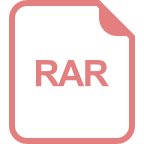
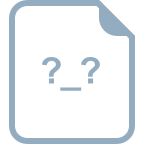
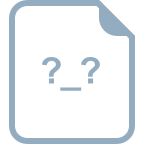
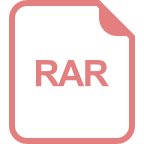
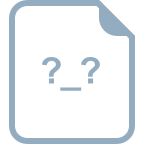
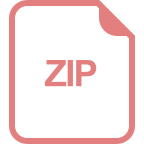
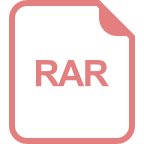
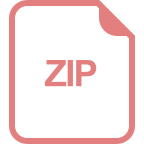