LocalDateTime.of(yesterday, time)
时间: 2023-11-02 07:59:06 浏览: 98
您想使用Java中的LocalDateTime类创建一个表示昨天某个时间的对象,是这样吗?要创建这样的对象,您可以使用以下代码:
```
LocalDate yesterday = LocalDate.now().minusDays(1);
LocalTime time = LocalTime.of(10, 30); // 假设时间是10:30
LocalDateTime dateTime = LocalDateTime.of(yesterday, time);
```
这将创建一个表示昨天10:30的LocalDateTime对象。请注意,上述代码假设您的系统时区为默认时区。如果您希望使用特定时区,请使用相应的`ZoneId`参数传递给`LocalDateTime.of()`方法。例如:
```
ZoneId zone = ZoneId.of("Asia/Shanghai"); // 设置为上海时区
LocalDateTime dateTime = LocalDateTime.of(yesterday, time, zone);
```
希望能对您有所帮助!如果您有任何其他问题,请随时提问。
相关问题
java.time.LocalDateTime的用法
Java的java.time.LocalDateTime类可以表示日期和时间,包括年、月、日、小时、分钟、秒和纳秒。
以下是java.time.LocalDateTime类的使用示例:
1. 创建一个LocalDateTime实例:
```
LocalDateTime now = LocalDateTime.now();
```
2. 通过指定的年、月、日、小时、分钟和秒创建一个LocalDateTime实例:
```
LocalDateTime dateTime = LocalDateTime.of(2021, 9, 22, 12, 30, 0);
```
3. 获取LocalDateTime实例的年、月、日、小时、分钟和秒:
```
int year = dateTime.getYear();
int month = dateTime.getMonthValue();
int day = dateTime.getDayOfMonth();
int hour = dateTime.getHour();
int minute = dateTime.getMinute();
int second = dateTime.getSecond();
```
4. 对LocalDateTime实例进行加减操作:
```
LocalDateTime tomorrow = now.plusDays(1);
LocalDateTime yesterday = now.minusDays(1);
```
5. 比较两个LocalDateTime实例的大小:
```
boolean isAfter = now.isAfter(dateTime);
boolean isBefore = now.isBefore(dateTime);
```
6. 格式化LocalDateTime实例:
```
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
String formattedDateTime = dateTime.format(formatter);
```
以上是java.time.LocalDateTime类的一些基本用法。
Java LocalDateTime
LocalDateTime是Java 8中引入的新的日期时间API,用于表示不带时区的日期和时间。它提供了更加直观和易用的日期时间操作方法,相比之前的Date和Calendar类,使用起来更加方便。
LocalDateTime的主要特点如下:
1. 不可变:LocalDateTime、LocalDate、LocalTime都是不可变对象,修改这些对象会返回一个新的副本,而不是在原对象上修改。
2. 带时区信息:LocalDateTime包含了年、月、日、时、分、秒、纳秒等信息,同时还带有时区信息,可以精确到毫秒级别。
3. 日期时间转换:LocalDateTime提供了丰富的方法来进行日期时间的转换,例如将LocalDateTime转换为Date、String等。
4. 日期时间操作:LocalDateTime提供了丰富的方法来进行日期时间的加减操作,例如加上或减去天数、小时数、分钟数等。
以下是一些常用的LocalDateTime操作示例:
```java
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public class LocalDateTimeDemo {
public static void main(String[] args) {
// 获取当前时间
LocalDateTime now = LocalDateTime.now();
System.out.println("当前时间:" + now);
// 格式化时间
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss"); String formattedNow = now.format(formatter);
System.out.println("格式化后的时间:" + formattedNow);
// 日期时间加减操作
LocalDateTime tomorrow = now.plusDays(1);
System.out.println("明天的时间:" + tomorrow);
LocalDateTime yesterday = now.minusDays(1);
System.out.println("昨天的时间:" + yesterday);
LocalDateTime sameDay = now.withHour(12).withMinute(0).withSecond(0);
System.out.println("中午12点整的时间:" + sameDay);
}
}
```<span class="em">1</span><span class="em">2</span><span class="em">3</span><span class="em">4</span>
阅读全文
相关推荐
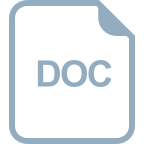
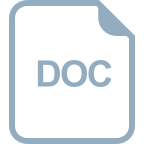
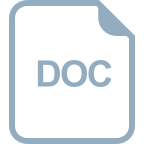





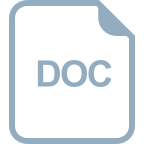
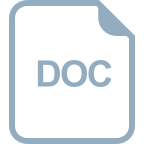
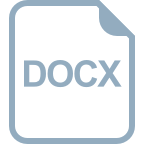
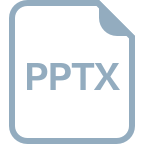
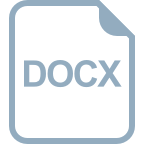
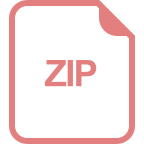
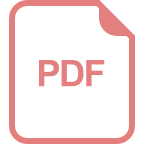
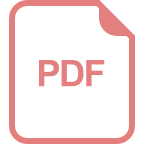
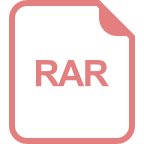