C语言 有一个字符串,包含n个字符。写一函数,将此字符串中从第m个字符开始的全部字符复制成另一个字符串
时间: 2024-11-22 22:20:53 浏览: 21
在C语言中,你可以使用`strcpy()`函数来完成这个任务,但如果需要从指定位置开始复制,可以自定义一个函数,例如下面的示例:
```c
#include <stdio.h>
#include <string.h>
// 定义函数,传入源字符串、目标字符串以及起始索引
void copy_from_index(char* src, char* dest, int start_index) {
if (start_index >= 0 && start_index <= strlen(src) - 1) { // 检查索引是否有效
size_t num_chars = strlen(src) - start_index; // 计算需要复制的字符数
strncpy(dest, &src[start_index], num_chars); // 使用strncpy防止越界并保留null终止符
dest[num_chars] = '\0'; // 确保目标字符串结束
} else {
printf("Invalid index provided.\n");
}
}
int main() {
char source[100];
printf("Enter the source string: ");
fgets(source, sizeof(source), stdin);
source[strcspn(source, "\n")] = '\0'; // 移除fgets读取的换行符
char target[100];
int m;
printf("Enter the starting index (1-based): ");
scanf("%d", &m);
copy_from_index(source, target, m - 1); // 函数调用时,索引需减1,因为数组索引从0开始计数
printf("Copied substring: %s\n", target);
return 0;
}
```
在这个程序中,`copy_from_index`函数接受源字符串`src`、目标字符串`dest`和起始索引`start_index`。它首先检查索引的有效性,然后计算需要复制的字符数,并使用`strncpy`函数安全地复制字符到`dest`。注意,在实际应用中,你可能还需要处理错误情况,比如内存分配不足等。
阅读全文
相关推荐
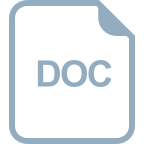
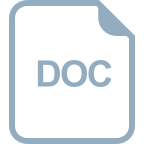
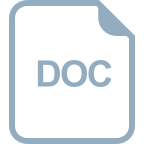
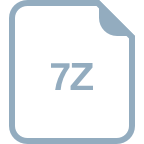
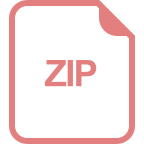
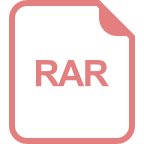
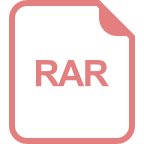
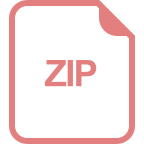
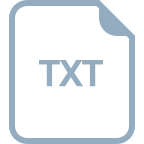
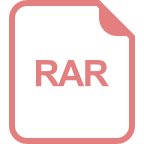
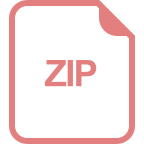
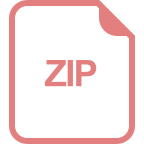
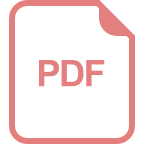
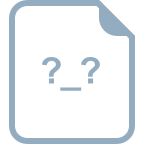
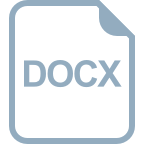
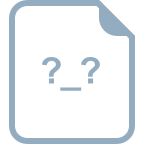
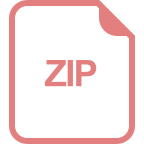
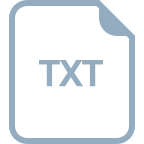