在Visual Studio 2019中读写yml文件的C++程序
时间: 2024-09-14 11:08:32 浏览: 64
在 Visual Studio 2019 中使用 C++ 来读写 YAML 文件,你可以利用第三方库如 yaml-cpp 或 ruamel.yaml。以下是简要步骤:
**使用yaml-cpp库:**
1. 首先,你需要在项目中添加yaml-cpp的依赖。你可以在CMakeLists.txt中配置或者直接从GitHub下载并安装。官网地址:https://github.com/jbeder/yaml-cpp
```cpp
cmake_minimum_required(VERSION 3.10)
find_package(yaml-cpp REQUIRED) # 搜索yaml-cpp库
```
2. 然后,在代码中读写YAML文件:
```cpp
#include <yaml-cpp/yaml.h>
// 读取YAML文件
std::ifstream file("config.yml");
if (!file.is_open()) {
std::cerr << "Failed to open file" << std::endl;
return;
}
yaml::Node node;
node.load(file);
auto config = node["section"]["key"]; // 根据实际YAML结构获取值
// 示例:存储字符串
yaml::Emitter emitter;
emitter << yaml::mappingBegin << "key": "value" << yaml::mappingEnd;
file << emitter.c_str();
file.close();
```
**使用ruamel.yaml库:**
ruamel.yaml比yaml-cpp更现代化,语法更接近Python。首先需要下载并安装它,然后操作类似:
```cpp
#include <ruyaml.hpp>
// 读取YAML文件
ruyaml::yaml::Loader loader;
std::string content = "";
std::ifstream file("config.yml");
if (!file) {
std::cerr << "Failed to open file" << std::endl;
return;
}
content.assign((std::istreambuf_iterator<char>(file)), std::istreambuf_iterator<char>());
ruyaml::Node node = loader.load(content);
// 获取数据
auto value = node["section"]["key"].as<std::string>();
// 写入YAML文件
ruyaml::Emitter emitter;
emitter << node << std::endl;
file << emitter.c_str();
file.close();
```
阅读全文
相关推荐
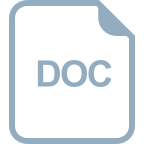
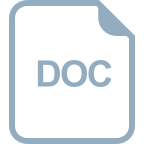
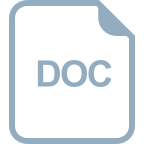
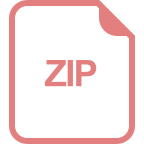
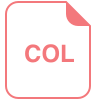
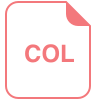
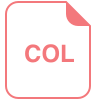
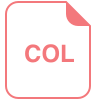
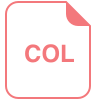
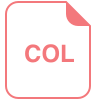
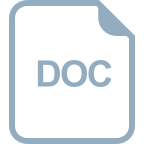
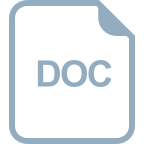
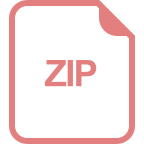
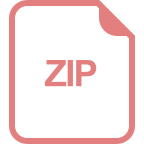