写一个输入一个十进制数转换成二进制数,并且转成其二进制数的补码的代码C语言
时间: 2024-12-20 12:16:21 浏览: 4
在C语言中,你可以通过以下步骤编写一个函数来将十进制数转换为二进制数,并计算其补码形式:
```c
#include <stdio.h>
#include <limits.h>
// Function to convert decimal to binary
void decimalToBinary(int num) {
int binaryNum[32], i = 0;
if (num == 0)
printf("Binary representation of 0 is 0\n");
else {
while (num > 0) {
binaryNum[i] = num % 2;
num /= 2;
i++;
}
// Print the binary representation in reverse order
for (int j = i - 1; j >= 0; j--)
printf("%d", binaryNum[j]);
printf("\n");
}
// Calculate and print the two's complement of the binary number
int twoComplement = 1 << (sizeof(int) * 8 - 1); // Assuming an int is 32 bits
if (binaryNum[0] == 1)
printf("Two's complement (negative): ");
else
printf("Two's complement (positive): ");
for (i = 0; i < sizeof(int) * 8; i++) {
printf("%d", (twoComplement ^ binaryNum[i]) & ~twoComplement);
}
printf("\n");
}
int main() {
int decimalInput;
printf("Enter a decimal number: ");
scanf("%d", &decimalInput);
decimalToBinary(decimalInput);
return 0;
}
```
在这个代码里,`decimalToBinary` 函数接收一个整数,将其转换为二进制并显示出来。然后,它会计算该二进制数的补码表示,并展示结果。
注意:这个代码假设输入的数字适合于32位整型。如果需要处理其他位宽,你需要调整`sizeof(int)`部分。
阅读全文
相关推荐
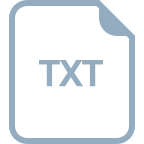
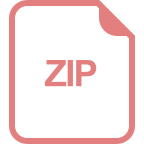
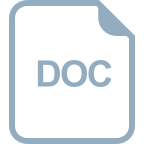















