c语言变量自加串口打印输出
时间: 2024-09-08 22:04:08 浏览: 116
在C语言中,如果你想要通过串口打印输出变量的自增操作,首先你需要设置好串口通信相关的硬件配置,并利用某个库(如`stdio.h`、`serial_port.h`等)提供的函数来处理串口通信。
假设有一个整数变量`count`,你可以这样做:
```c
#include <stdio.h>
#include <serial_port.h> // 假设这是串口库
int count = 0;
void print_and_increment() {
// 打印当前计数值到串口
printf("Current count: %d\n", count);
// 自加操作
count++;
// 写入串口
write_to_uart(count); // 调用串口函数将count的值发送出去
}
int main() {
// 初始化串口
initialize_uart();
// 循环打印并自增
while (1) {
print_and_increment();
delay_ms(1000); // 暂停一段时间,以便观察输出
}
return 0;
}
```
在这个例子中,你需要实现`write_to_uart()`函数来实际发送数据到串口,`initialize_uart()`用于初始化串口通信。注意,具体的库函数和功能可能会有所不同,这个只是一个基本的概念。
相关问题
写一段C语言用通过UART串口printf输出变量内容
可以使用标准 C 库函数 printf,并在 UART 串口上输出。
举个例子,假设有一个整型变量 `a`,要在 UART 串口上输出它的值,可以使用以下代码:
```
#include <stdio.h>
int main()
{
int a = 123;
printf("a = %d\n", a);
return 0;
}
```
在这段代码中,%d 是用来格式化输出整型变量的占位符。回车换行符 `\n` 表示输出完这行后换行。
你也可以使用其他占位符来格式化输出其他类型的变量,例如:
- %c 输出字符型变量
- %f 输出浮点型变量
- %s 输出字符串型变量
你可以参考这份文档了解更多关于 printf 函数的用法:http://www.cplusplus.com/reference/cstdio/printf/
希望这能帮到你!
写一段C语言用通过UART串口输出变量内容
好的,下面是一段在 C 语言中使用 UART 串口输出变量内容的代码片段:
```
#include <stdio.h>
#include <string.h>
int main(void) {
int x = 123;
char str[10];
// 将 x 的值转换为字符串
sprintf(str, "%d", x);
// 通过 UART 串口输出字符串
uart_puts(str);
return 0;
}
```
在上面的代码中,我们使用了 `sprintf` 函数将整数 `x` 转换为字符串,然后使用自定义的 `uart_puts` 函数通过 UART 串口输出字符串。
注意:上述代码中的 `uart_puts` 函数需要你自己实现。
阅读全文
相关推荐
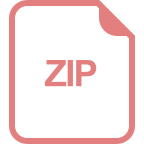
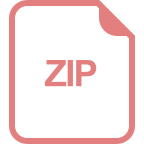
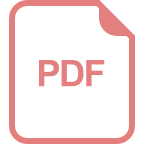
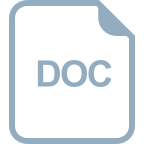
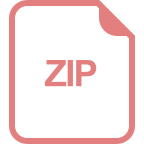
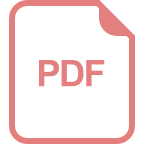
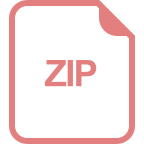
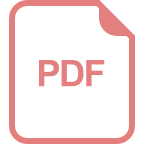
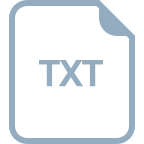
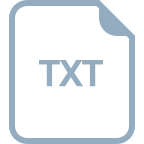
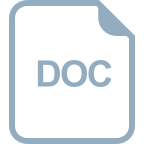
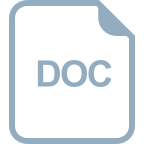
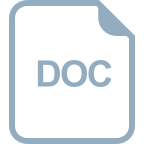
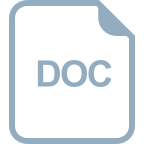
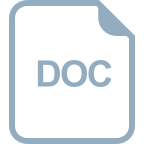

