C# 使png图片的白色部分透明
时间: 2024-10-13 19:07:18 浏览: 43
在C#中,你可以使用`System.Drawing.Imaging`命名空间下的`Bitmap`类以及一些图像处理函数来将PNG图片的白色部分变为透明。这里是一个简单的示例:
```csharp
using System.Drawing;
using System.Drawing.Imaging;
public Bitmap MakeTransparent(Bitmap image)
{
// 创建一个新的Alpha通道Bitmap
using (var transparentBitmap = new Bitmap(image.Width, image.Height, PixelFormat.Format32bppArgb))
{
// 获取Graphics对象
using (var graphics = Graphics.FromImage(transparentBitmap))
{
// 设置颜色填充模式为透明
graphics.CompositingMode = CompositingMode.SourceCopy;
// 遍历图片的每个像素
for (int x = 0; x < image.Width; x++)
for (int y = 0; y < image.Height; y++)
{
// 检查当前像素是否为白色
Color color = image.GetPixel(x, y);
if (!color.A > 0 || !IsWhite(color)) // 判断像素是不是纯白
{
transparentBitmap.SetPixel(x, y, color); // 如果不是白色,则复制到新的Bitmap
}
}
}
return transparentBitmap;
}
}
private bool IsWhite(Color color)
{
// 可能的白色阈值判断,可以根据实际需求调整
const int WhiteThreshold = 255;
return color.R == WhiteThreshold && color.G == WhiteThreshold && color.B == WhiteThreshold;
}
```
这段代码会创建一个新的Bitmap,然后遍历原图像中的每一个像素,如果像素颜色不是纯白色,就将其复制到新Bitmap上,使其透明。`IsWhite`函数用于检查颜色是否足够接近白色。
使用此方法前,请确保已引用了`System.Drawing`库。此外,这种方法可能会对性能造成影响,尤其是对于大尺寸图片。如果性能至关重要,可以考虑使用专门的图像处理库,如AForge.NET或SixLabors.ImageSharp。
阅读全文
相关推荐
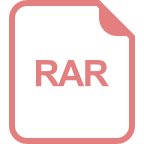
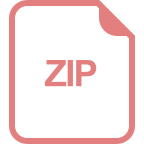
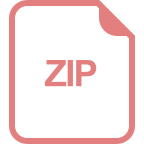

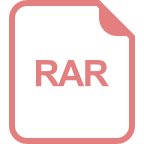
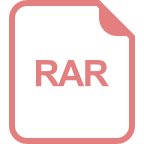
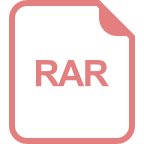
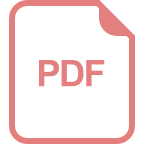
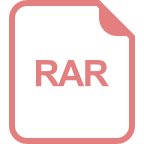
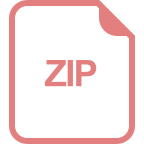
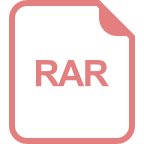
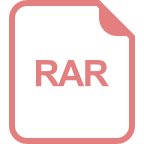
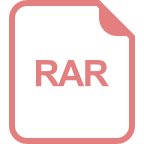
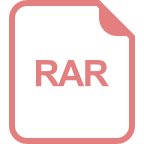
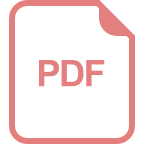
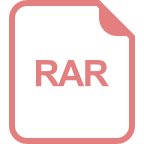
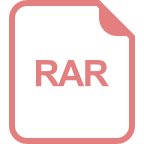
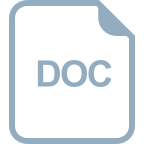
